如何在lightweight OpenPose人体姿态估计网络中添加SENet注意力模块,代码实现
时间: 2023-06-12 15:02:39 浏览: 63
在lightweight OpenPose网络中添加SENet注意力模块的步骤如下:
1. 导入必要的库
``` python
import torch
import torch.nn as nn
```
2. 定义SENet注意力模块
``` python
class SELayer(nn.Module):
def __init__(self, in_channels, reduction_ratio=16):
super(SELayer, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool2d(1)
self.fc = nn.Sequential(
nn.Linear(in_channels, in_channels // reduction_ratio),
nn.ReLU(inplace=True),
nn.Linear(in_channels // reduction_ratio, in_channels),
nn.Sigmoid()
)
def forward(self, x):
b, c, _, _ = x.size()
y = self.avg_pool(x).view(b, c)
y = self.fc(y).view(b, c, 1, 1)
return x * y.expand_as(x)
```
3. 在lightweight OpenPose网络中添加SENet注意力模块
``` python
class ConvBlock(nn.Module):
def __init__(self, in_channels, out_channels):
super(ConvBlock, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.bn1 = nn.BatchNorm2d(out_channels)
self.conv2 = nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.bn2 = nn.BatchNorm2d(out_channels)
self.se = SELayer(out_channels)
def forward(self, x):
out = self.conv1(x)
out = F.relu(self.bn1(out))
out = self.conv2(out)
out = F.relu(self.bn2(out))
out = self.se(out) # 添加SENet注意力模块
return out
class LightweightOpenPose(nn.Module):
def __init__(self, in_channels, num_keypoints):
super(LightweightOpenPose, self).__init__()
self.conv1_1 = nn.Conv2d(in_channels, 64, kernel_size=3, stride=1, padding=1)
self.bn1_1 = nn.BatchNorm2d(64)
self.conv1_2 = nn.Conv2d(64, 64, kernel_size=3, stride=1, padding=1)
self.bn1_2 = nn.BatchNorm2d(64)
self.pool1 = nn.MaxPool2d(kernel_size=2, stride=2)
self.conv2_1 = ConvBlock(64, 128)
self.pool2 = nn.MaxPool2d(kernel_size=2, stride=2)
self.conv3_1 = ConvBlock(128, 256)
self.conv3_2 = ConvBlock(256, 256)
self.conv3_3 = ConvBlock(256, 256)
self.conv3_4 = ConvBlock(256, 256)
self.conv4_1 = ConvBlock(256, 512)
self.conv4_2 = ConvBlock(512, 512)
self.conv4_3_CPM = ConvBlock(512, 128)
self.conv4_3_CPM_bn = nn.BatchNorm2d(128)
self.conv4_4_CPM = nn.Conv2d(128, num_keypoints, kernel_size=1, stride=1, padding=0)
def forward(self, x):
out = F.relu(self.bn1_1(self.conv1_1(x)))
out = F.relu(self.bn1_2(self.conv1_2(out)))
out = self.pool1(out)
out = self.conv2_1(out)
out = self.pool2(out)
out = self.conv3_1(out)
out = self.conv3_2(out)
out = self.conv3_3(out)
out = self.conv3_4(out)
out = self.conv4_1(out)
out = self.conv4_2(out)
out = F.relu(self.conv4_3_CPM_bn(self.conv4_3_CPM(out)))
out = self.conv4_4_CPM(out)
return out
```
这样就完成了在lightweight OpenPose网络中添加SENet注意力模块的代码实现。
相关推荐
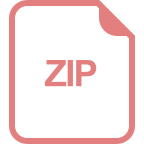
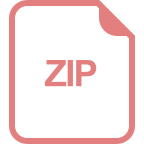














