前端vue调用websocket接口
时间: 2023-10-19 14:07:50 浏览: 60
在Vue中调用WebSocket接口,可以使用Websocket API。以下是一个简单的示例:
1. 在Vue组件中引入WebSocket:
```javascript
import io from 'socket.io-client'
```
2. 创建WebSocket连接:
```javascript
mounted () {
this.socket = io('ws://localhost:3000')
this.socket.on('connect', () => {
console.log('connected to server')
})
}
```
3. 发送和接收消息:
```javascript
methods: {
sendMessage () {
this.socket.emit('message', this.message)
}
},
created () {
this.socket.on('message', (data) => {
console.log(data)
})
}
```
这样,Vue组件就可以通过WebSocket与后端建立连接,发送和接收消息。
相关问题
前端vue脚手架调用websocket接口携带参数
在前端使用 Vue 脚手架调用 WebSocket 接口并携带参数的步骤下:
1. 在 Vue 组件中创建 WebSocket 连接:首先,在 Vue 组件中创建一个 WebSocket 实例,并在 `created`子函数中进行连接:
```javascript
import { mapState } from 'vuex';
export default {
data() {
return {
socket: null
};
},
created() {
this.socket = new WebSocket('ws://your-server-address');
this.socket.onopen = () => {
console.log('Connected to the WebSocket server');
// 连接成功后发送参数
const params = {
userId: this.userId,
token: this.token
// 其他参数
};
this.socket.send(JSON.stringify(params));
};
this.socket.onmessage = (event) => {
const message = JSON.parse(event.data);
console.log('Received message:', message);
// 处理接收到的消息
};
this.socket.onclose = () => {
console.log('Disconnected from the WebSocket server');
};
},
computed: {
...mapState(['userId', 'token'])
}
}
```
2. 在服务器端接收和处理参数:在服务器端,根据你使用的 WebSocket 库,可以监听连接事件并接收和处理参数。下面以 `ws` 库为例:
```javascript
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
console.log('A client connected');
ws.on('message', (message) => {
// 处理接收到的消息
const params = JSON.parse(message);
console.log('Received parameters:', params);
// 发送消息给客户端
ws.send('Hello, client!');
});
ws.on('close', () => {
console.log('A client disconnected');
});
});
```
在上述示例中,前端在连接成功后,会将参数以 JSON 字符串的形式发送给服务器端。服务器端接收到消息后,将其解析为参数,并进行相应处理。然后,服务器端可以向客户端发送消息。
请注意,上述示例中的参数发送方式仅为示例,并不具体指定 `userId` 和 `token` 的来源,你可以根据你的项目需求进行相应的调整。另外,还需确保 WebSocket 连接的地址、端口和参数的格式与你的实际情况相匹配。
websocket前端vue
对于前端使用 Vue 实现 WebSocket 的方式,可以按照以下步骤进行:
1. 首先,安装 WebSocket 的库。可以使用 npm 或 yarn 命令来安装 `vue-native-websocket` 或 `vue-socket.io` 等 WebSocket 相关的库。
2. 在 Vue 组件中引入 WebSocket 库。在需要使用 WebSocket 功能的组件中,通过 `import` 语句引入 WebSocket 库。
3. 在 Vue 组件中创建 WebSocket 实例。在 Vue 组件的 `created()` 或 `mounted()` 生命周期钩子函数中,通过实例化 WebSocket 对象来创建 WebSocket 连接,并设置相关的事件监听器。
4. 在 Vue 组件中处理 WebSocket 事件。可以在 WebSocket 连接建立成功、收到消息、连接关闭等事件发生时,通过回调函数来处理相应的逻辑。
5. 在 Vue 组件中发送消息。可以通过调用 WebSocket 实例的方法,如 `send()` 来发送消息给服务器。
需要注意的是,WebSocket 是基于事件驱动的,因此需要合理处理各种事件,并根据业务需求进行逻辑编写。以上是一个简单的步骤示例,具体实现可以根据具体的需求和所选择的 WebSocket 库进行调整。
相关推荐
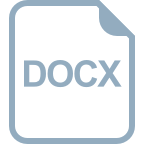
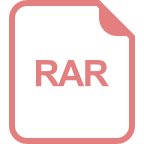












