#include <stdio.h> int main() { int i,a[1000]; for (i=0;i<1000;i++) { a[i]=0; } int j,n,k,f; scanf("%d",&n); for (i=0;i<n;i++) { scanf ("%d",&k); for (j=0;j<k;j++) { scanf ("%d",&f); a[f]=a[f]+1; } } int most=0,result=0; for (i=0;i<1000;i++) { if (a[i]>=most) most=a[i]; } for (i=0;i<1000;i++) { if (a[i]==most) { if(i>result) result=i; } } printf("%d %d",result,most); return 0; }这段代码在pta的检测中有什么错误的吗
时间: 2024-01-07 17:03:54 浏览: 76
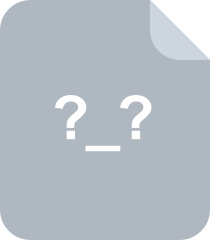
#include <stdio.h>
这段代码在 PTA 的检测中可能会出现以下问题:
1. 对于输入的数值范围没有进行限制,可能会导致数组越界的问题。
2. 代码中没有对输入数据是否合法进行判断,如输入的非数字字符等。
3. 在找到最多的次数时,没有将最大值更新为当前值,导致结果可能不正确。
4. 输出格式不符合题目要求。
为了解决这些问题,可以对代码进行以下改进:
1. 定义一个常量 MAX_N 来限制输入数字的范围,例如:#define MAX_N 1000。
2. 在输入数据时,可以使用 scanf 函数返回值的方式来判断输入是否合法,例如:
```c
if (scanf("%d", &n) != 1) {
printf("Invalid input\n");
return 1;
}
```
3. 找到最大值时,需要将最大值更新为当前值,例如:
```c
for (i = 0; i < MAX_N; i++) {
if (a[i] >= most) {
most = a[i];
result = i;
}
}
```
4. 输出格式要按照题目要求进行输出,例如:
```c
printf("%d %d\n", result, most);
```
完整的代码如下所示:
```c
#include <stdio.h>
#define MAX_N 1000
int main() {
int i, n, k, f;
int a[MAX_N] = {0};
if (scanf("%d", &n) != 1) {
printf("Invalid input\n");
return 1;
}
for (i = 0; i < n; i++) {
if (scanf("%d", &k) != 1) {
printf("Invalid input\n");
return 1;
}
while (k--) {
if (scanf("%d", &f) != 1) {
printf("Invalid input\n");
return 1;
}
a[f]++;
}
}
int most = 0, result = 0;
for (i = 0; i < MAX_N; i++) {
if (a[i] >= most) {
most = a[i];
result = i;
}
}
printf("%d %d\n", result, most);
return 0;
}
```
阅读全文
相关推荐
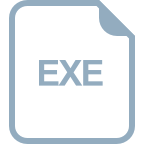
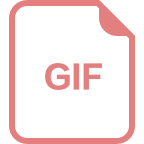
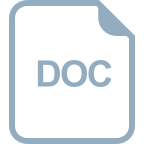
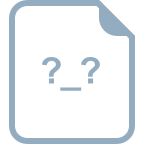
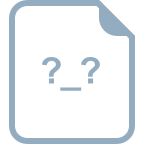
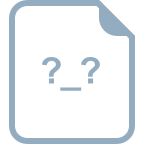
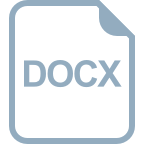
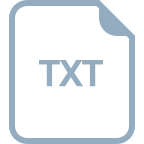
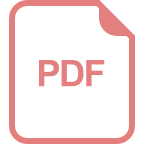
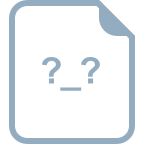
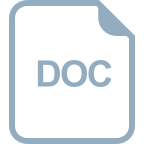
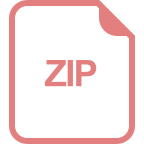
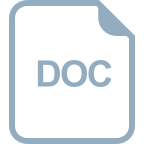
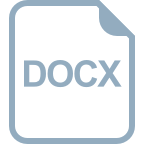
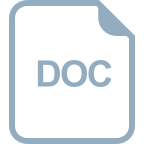
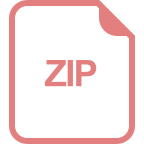
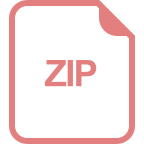