c语言读取串口数据的代码
时间: 2023-10-25 17:09:58 浏览: 158
当你使用C语言读取串口数据时,你可以使用标准的输入输出库函数来完成。以下是一个简单的示例代码,演示了如何读取串口数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main() {
int serial_port = open("/dev/ttyS0", O_RDWR);
if (serial_port < 0) {
printf("无法打开串口\n");
return -1;
}
struct termios tty;
memset(&tty, 0, sizeof(tty));
if (tcgetattr(serial_port, &tty) != 0) {
printf("无法获取串口属性\n");
return -1;
}
// 设置串口参数
tty.c_cflag &= ~PARENB; // 无奇偶校验
tty.c_cflag &= ~CSTOPB; // 1位停止位
tty.c_cflag &= ~CSIZE; // 清除数据位设置
tty.c_cflag |= CS8; // 8位数据位
tty.c_cflag &= ~CRTSCTS; // 禁用硬件流控制
tty.c_cflag |= CREAD | CLOCAL; // 启用接收和本地模式
tty.c_iflag &= ~(IXON | IXOFF | IXANY); // 禁用软件流控制
tty.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG); // 设置为原始模式
tty.c_oflag &= ~OPOST; // 设置为原始模式
tty.c_cc[VMIN] = 0; // 读取最小字符数
tty.c_cc[VTIME] = 10; // 读取超时时间(单位:0.1秒)
if (cfsetispeed(&tty, B9600) != 0 || cfsetospeed(&tty, B9600) != 0) {
printf("无法设置串口波特率\n");
return -1;
}
if (tcsetattr(serial_port, TCSANOW, &tty) != 0) {
printf("无法设置串口属性\n");
return -1;
}
while (1) {
unsigned char buffer[256];
int num_bytes = read(serial_port, buffer, sizeof(buffer));
if (num_bytes > 0) {
for (int i = 0; i < num_bytes; i++) {
printf("%c", buffer[i]);
}
fflush(stdout);
}
}
close(serial_port);
return 0;
}
```
请注意,代码中使用的串口设备路径 `/dev/ttyS0` 是Linux系统中默认的串口设备路径。如果你的系统中串口设备路径不同,请根据实际情况进行修改。此外,代码中的波特率设置为9600,你也可以根据需要进行修改。
希望对你有帮助!如果有更多问题,请随时提问。
相关推荐
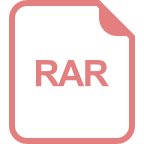
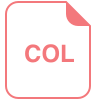
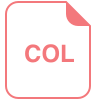
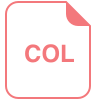











