python ctypes获取Linux ipc的共享内存,并写入数据
时间: 2024-05-08 15:21:59 浏览: 16
要使用Python的ctypes模块获取Linux的IPC共享内存并写入数据,您需要以下步骤:
1. 导入ctypes模块
```python
import ctypes
```
2. 使用ctypes库中的库函数获取共享内存
```python
libc = ctypes.CDLL('libc.so.6')
shmget = libc.shmget
shmat = libc.shmat
```
3. 调用shmget函数获取共享内存
```python
shm_id = shmget(key, size, 0o666 | IPC_CREAT)
```
其中,key是IPC键,size是共享内存大小,0o666是权限位,IPC_CREAT是标志位,表示如果共享内存不存在就创建一个新的共享内存。
4. 调用shmat函数将共享内存连接到进程空间
```python
shm_addr = shmat(shm_id, 0, 0)
```
其中,shm_id是共享内存ID,0表示让内核自动选择内存地址,0表示没有特殊操作。
5. 将数据写入共享内存
```python
data = "Hello World"
ctypes.memmove(shm_addr, data.encode('utf-8'), len(data.encode('utf-8')))
```
其中,data是要写入的数据,使用memmove函数将数据写入共享内存。
6. 分离共享内存
```python
libc.shmdt(shm_addr)
```
最后,使用shmdt函数分离共享内存。
完整的Python代码示例:
```python
import ctypes
libc = ctypes.CDLL('libc.so.6')
shmget = libc.shmget
shmat = libc.shmat
key = 1234
size = 1024
shm_id = shmget(key, size, 0o666 | IPC_CREAT)
shm_addr = shmat(shm_id, 0, 0)
data = "Hello World"
ctypes.memmove(shm_addr, data.encode('utf-8'), len(data.encode('utf-8')))
libc.shmdt(shm_addr)
```
相关推荐
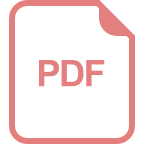
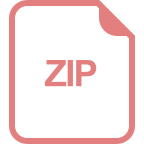
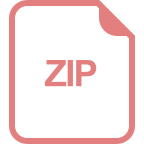














