解释void InOrderTraverse(BiTree T) { /*中序遍历*/ if (T) { InOrderTraverse(T->lchild); cout << T->data; InOrderTraverse(T->rchild); } }
时间: 2023-06-07 08:06:47 浏览: 121
这个函数是一个二叉树的中序遍历函数,它按照 左孩子 -> 根节点 -> 右孩子 的顺序遍历二叉树。其中,参数T是一个二叉树结构体的指针。在函数体内部,先判断当前节点是否为空,如果不为空,就对它的左子树进行递归中序遍历,然后输出当前节点的数据,最后对右子树进行递归中序遍历。这个函数的作用是打印出二叉树的节点数据(键值),并且按照从小到大的顺序输出。
相关问题
#include<iostream> using namespace std; typedef struct BiTNode { int data; int cnt; struct BiTNode *lchild,*rchild; }BiTNode,*BiTree; int sum=0; //全局变量sum表示排序树当前结点的个数,也为了判断数据是否为输出的最后一个数 void SearchBST(BiTree &T,int x) {//基于非递归的二叉排序树的结点的查找和插入 } void InOrderTraverse(BiTree T) {//中序遍历输出二叉树T结点 } void Print_Count(BiTree T,int x) {//中序遍历输出二叉树T计数 },补充我的代码
以下是补充完整的代码,其中包括了基于非递归的二叉排序树的结点的查找和插入、中序遍历输出二叉树结点以及中序遍历输出二叉树计数的实现。你可以根据自己的需求进行调整。
```
#include<iostream>
using namespace std;
typedef struct BiTNode {
int data;
int cnt;
struct BiTNode *lchild,*rchild;
}BiTNode,*BiTree;
int sum=0; //全局变量sum表示排序树当前结点的个数,也为了判断数据是否为输出的最后一个数
void SearchBST(BiTree &T,int x) {//基于非递归的二叉排序树的结点的查找和插入
if(T==NULL) {//如果根节点为空,则新建节点作为根节点
T=new BiTNode;
T->data=x;
T->cnt=1;
T->lchild=NULL;
T->rchild=NULL;
} else {//否则遍历树,找到合适的位置进行插入或计数
BiTree p=T;
while(p!=NULL) {
if(p->data==x) {
p->cnt++;
break;
} else if(p->data>x) {
if(p->lchild==NULL) {
BiTree q=new BiTNode;
q->data=x;
q->cnt=1;
q->lchild=NULL;
q->rchild=NULL;
p->lchild=q;
break;
} else {
p=p->lchild;
}
} else {
if(p->rchild==NULL) {
BiTree q=new BiTNode;
q->data=x;
q->cnt=1;
q->lchild=NULL;
q->rchild=NULL;
p->rchild=q;
break;
} else {
p=p->rchild;
}
}
}
}
}
void InOrderTraverse(BiTree T) {//中序遍历输出二叉树T结点
if(T==NULL) {
return;
}
InOrderTraverse(T->lchild);
cout<<T->data<<" ";
InOrderTraverse(T->rchild);
}
void Print_Count(BiTree T,int x) {//中序遍历输出二叉树T计数
if(T==NULL) {
return;
}
Print_Count(T->lchild,x);
if(T->data==x) {
cout<<T->cnt<<endl;
sum++;
}
if(sum==T->cnt) {//判断数据是否为输出的最后一个数
return;
}
Print_Count(T->rchild,x);
}
int main() {
BiTree T=NULL;//初始化二叉排序树
int n,m;
cin>>n>>m;
for(int i=0;i<n;i++) {
int x;
cin>>x;
SearchBST(T,x);//向二叉排序树中插入数据
}
InOrderTraverse(T);//中序遍历输出二叉树结点
cout<<endl;
for(int i=0;i<m;i++) {
int x;
cin>>x;
sum=0;//初始化sum
Print_Count(T,x);//中序遍历输出数据x的计数
}
return 0;
}
```
#include <bits/stdc++.h> using namespace std; typedef string ElemType; typedef struct BitNode { ElemType data; struct BitNode *lchild,*rchild; } BitNode,*BiTree; //char sc[10]= {"+-*/"}; void CreateBiTree(BiTree &t) { string ch; cin >> ch; if(ch[0] == '#') t = NULL; else { t = new BitNode; t->data = ch; CreateBiTree(t->lchild); CreateBiTree(t->rchild); } } void InOrderTraverse(BiTree t) { if(t) { string op = t->data; if(op[0]<'0' || op[0]>'9') cout << '('; InOrderTraverse(t->lchild); cout << t->data ; InOrderTraverse(t->rchild); if(op[0]<'0' || op[0]>'9') cout << ')'; } /***********************************/ } int main() { BiTree tree = new BitNode; string ch; while(cin >> ch) { tree->data = ch; CreateBiTree(tree->lchild); CreateBiTree(tree->rchild); InOrderTraverse(tree); printf("\n"); } return 0; }
这段代码是一个表达式树的建立和遍历程序,可以读入一条表达式,以二叉树的形式建立表达式树,并按照中序遍历的顺序输出中缀表达式。具体实现过程是先读入一个字符串作为根节点的值,然后递归读入左子树和右子树,再按照中序遍历的顺序遍历树并输出表达式。其中,如果遍历到运算符节点,则输出括号,以保证正确的优先级顺序。
阅读全文
相关推荐
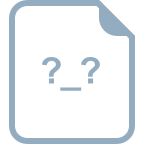
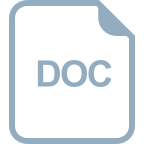
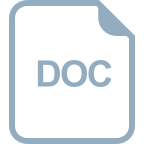




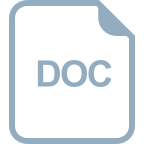
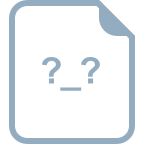

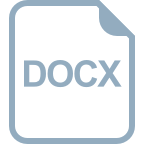
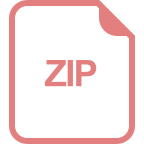
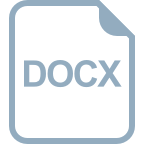