Write the code about Readers-Writer Problem Implementation in C++.1、 A data set is shared among several concurrent processes: Readers – only read the data set; they do not perform any updates. Writers – can both read and write. 2、 Problem – allow multiple readers (at most 8) to read at the same time. Only one single writer can access the shared data at the same time
时间: 2024-01-27 18:03:49 浏览: 80
Here is an example implementation of the Readers-Writer Problem in C++ using semaphores:
```cpp
#include <iostream>
#include <thread>
#include <chrono>
#include <semaphore.h>
using namespace std;
// Initialize Semaphores
sem_t rw_mutex, mutex;
int read_count = 0;
// Writer Function
void writer(int id) {
while (true) {
// Wait for Writer Semaphore
sem_wait(&rw_mutex);
// Write to Data Set
cout << "Writer " << id << " is writing to the data set." << endl;
this_thread::sleep_for(chrono::milliseconds(1000));
// Release Writer Semaphore
sem_post(&rw_mutex);
}
}
// Reader Function
void reader(int id) {
while (true) {
// Wait for Mutex Semaphore
sem_wait(&mutex);
// Increment Read Count
read_count++;
// If First Reader, Wait for Writer Semaphore
if (read_count == 1) {
sem_wait(&rw_mutex);
}
// Release Mutex Semaphore
sem_post(&mutex);
// Read from Data Set
cout << "Reader " << id << " is reading from the data set." << endl;
this_thread::sleep_for(chrono::milliseconds(500));
// Wait for Mutex Semaphore
sem_wait(&mutex);
// Decrement Read Count
read_count--;
// If Last Reader, Release Writer Semaphore
if (read_count == 0) {
sem_post(&rw_mutex);
}
// Release Mutex Semaphore
sem_post(&mutex);
}
}
int main() {
// Initialize Semaphores
sem_init(&rw_mutex, 0, 1);
sem_init(&mutex, 0, 1);
// Create Writer Threads
thread writer1(writer, 1);
// Create Reader Threads
thread reader1(reader, 1);
thread reader2(reader, 2);
thread reader3(reader, 3);
thread reader4(reader, 4);
thread reader5(reader, 5);
thread reader6(reader, 6);
thread reader7(reader, 7);
thread reader8(reader, 8);
// Join Threads
writer1.join();
reader1.join();
reader2.join();
reader3.join();
reader4.join();
reader5.join();
reader6.join();
reader7.join();
reader8.join();
// Destroy Semaphores
sem_destroy(&rw_mutex);
sem_destroy(&mutex);
return 0;
}
```
In this implementation, we use two semaphores: `rw_mutex` and `mutex`. The `rw_mutex` semaphore controls access to the shared data set for writers, while the `mutex` semaphore controls access to the `read_count` variable for readers.
When a writer wants to access the shared data set, it waits for the `rw_mutex` semaphore to be available, writes to the data set, and then releases the `rw_mutex` semaphore.
When a reader wants to access the shared data set, it waits for the `mutex` semaphore to be available, increments the `read_count` variable, and if it's the first reader, waits for the `rw_mutex` semaphore to be available. It then reads from the data set, decrements the `read_count` variable, and if it's the last reader, releases the `rw_mutex` semaphore. Finally, it releases the `mutex` semaphore.
We create one writer thread and eight reader threads as an example, but the number of readers can be adjusted as needed.
阅读全文
相关推荐
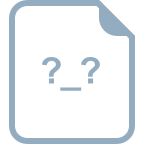
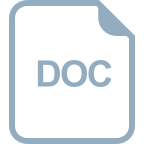


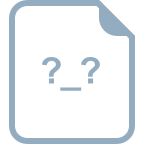
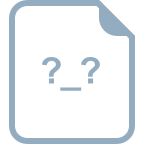
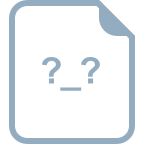
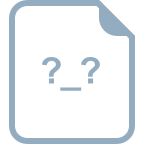
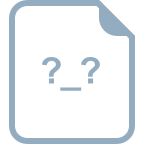
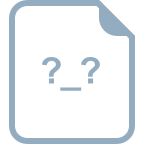
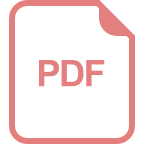
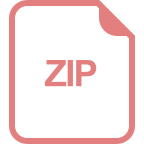