Write the code about Readers-Writer Problem Implementation.1、 A data set is shared among several concurrent processes: Readers – only read the data set; they do not perform any updates. Writers – can both read and write. 2、 Problem – allow multiple readers (at most 8) to read at the same time. Only one single writer can access the shared data at the same time
时间: 2023-12-06 18:45:11 浏览: 25
Here is an implementation of the Readers-Writer problem in Python, using semaphores:
```python
import threading
class ReadersWriterLock:
def __init__(self):
self.lock = threading.Lock()
self.readers_sem = threading.Semaphore(8)
self.writers_sem = threading.Semaphore(1)
self.read_count = 0
def acquire_read(self):
with self.lock:
self.read_count += 1
if self.read_count == 1:
self.writers_sem.acquire()
self.readers_sem.acquire()
def release_read(self):
self.readers_sem.release()
with self.lock:
self.read_count -= 1
if self.read_count == 0:
self.writers_sem.release()
def acquire_write(self):
self.writers_sem.acquire()
def release_write(self):
self.writers_sem.release()
```
This implementation uses two semaphores - one for readers and one for writers. The `readers_sem` semaphore has a maximum count of 8, allowing up to 8 readers to hold the semaphore at the same time. The `writers_sem` semaphore has a maximum count of 1, allowing only one writer to hold the semaphore at a time.
When a reader wants to read the shared data, it first acquires the `readers_sem` semaphore, which allows up to 8 readers to hold it concurrently. It also increments the `read_count` variable, which keeps track of how many readers are currently reading the data. If this is the first reader, the reader also acquires the `writers_sem` semaphore to prevent any writers from accessing the data while it is being read.
When a reader is done reading the data, it releases the `readers_sem` semaphore and decrements the `read_count` variable. If this was the last reader, the reader also releases the `writers_sem` semaphore to allow any waiting writers to access the data.
When a writer wants to write to the shared data, it first acquires the `writers_sem` semaphore, which allows only one writer to hold it at a time. This prevents any other readers or writers from accessing the data while it is being written to.
When the writer is done writing to the data, it releases the `writers_sem` semaphore to allow any waiting readers or writers to access the data.
相关推荐
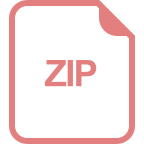
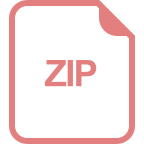















