pthread_join例子
时间: 2023-08-03 15:42:38 浏览: 86
下面是一个简单的 pthread_join 的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *thread_func(void *arg)
{
int *p = (int *)arg;
printf("Thread %d is running.\n", *p);
pthread_exit(NULL);
}
int main()
{
pthread_t tid;
int arg = 1;
int ret = pthread_create(&tid, NULL, thread_func, (void *)&arg);
if (ret != 0) {
perror("pthread_create");
exit(EXIT_FAILURE);
}
printf("Thread %d is created.\n", arg);
ret = pthread_join(tid, NULL);
if (ret != 0) {
perror("pthread_join");
exit(EXIT_FAILURE);
}
printf("Thread %d is terminated.\n", arg);
return 0;
}
```
该程序创建了一个线程,线程的入口函数是 thread_func,该函数接收一个参数,将其转换成 int 类型,打印出线程的编号,然后退出。
在主线程中,首先创建了一个线程,然后打印出线程的编号,接着调用 pthread_join 等待该线程退出,最后打印出该线程的编号。
需要注意的是,pthread_join 的第二个参数是一个 void ** 类型的指针,用于获取线程的返回值,如果不需要获取,则可以传入 NULL。在本例中,线程的返回值为 NULL。
阅读全文
相关推荐







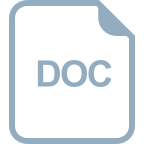







