举一个pthread_cond_wait例子
时间: 2023-10-17 13:13:13 浏览: 37
好的,以下是一个pthread_cond_wait的例子:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
int count = 0;
pthread_mutex_t mutex;
pthread_cond_t cond;
void *thread1(void *);
void *thread2(void *);
int main(int argc, char **argv) {
pthread_t t_a;
pthread_t t_b;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
pthread_create(&t_a, NULL, thread1, NULL);
pthread_create(&t_b, NULL, thread2, NULL);
pthread_join(t_a, NULL);
pthread_join(t_b, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
exit(0);
}
void *thread1(void *arg) {
pthread_mutex_lock(&mutex);
while (count < 10) {
if (count % 2 == 0) {
printf("count=%d\n", count);
count++;
pthread_cond_signal(&cond);
} else {
pthread_cond_wait(&cond, &mutex);
}
}
pthread_mutex_unlock(&mutex);
return NULL;
}
void *thread2(void *arg) {
pthread_mutex_lock(&mutex);
while (count < 10) {
if (count % 2 == 1) {
printf("count=%d\n", count);
count++;
pthread_cond_signal(&cond);
} else {
pthread_cond_wait(&cond, &mutex);
}
}
pthread_mutex_unlock(&mutex);
return NULL;
}
```
这个例子使用了pthread_mutex_t和pthread_cond_t两个同步机制来确保两个线程按照指定的顺序执行。两个线程都在一个while循环中等待,当先前的线程发出pthread_cond_signal()信号时才会继续执行。在此例中,thread1线程打印并增加count的值,当count的值是偶数时,该线程发出pthread_cond_signal()信号,使得thread2线程继续执行;反之,则thread1线程等待。
这个例子可能不是非常实际,但它可以帮助你理解pthread_cond_wait和pthread_cond_signal的工作原理。
相关推荐
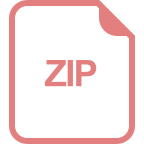














