pthread如何挂起一个任务
时间: 2023-09-11 17:11:24 浏览: 55
在 pthread 中,可以使用 pthread_cond_wait 函数来挂起一个任务,直到满足特定条件才会被唤醒。pthread_cond_wait 函数需要一个互斥锁 mutex 和一个条件变量 condvar,它的原型如下:
```c
int pthread_cond_wait(pthread_cond_t *condvar, pthread_mutex_t *mutex);
```
当一个线程调用 pthread_cond_wait 函数时,它会首先释放 mutex,然后等待另一个线程调用 pthread_cond_signal 或 pthread_cond_broadcast 函数来唤醒它。当线程被唤醒后,它会重新获得 mutex,并从 pthread_cond_wait 函数返回。
下面是一个简单的例子,演示了如何使用 pthread_cond_wait 函数挂起一个任务:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t condvar = PTHREAD_COND_INITIALIZER;
void *thread_func(void *arg) {
printf("Thread started.\n");
pthread_mutex_lock(&mutex);
printf("Thread locked mutex.\n");
pthread_cond_wait(&condvar, &mutex);
printf("Thread woken up.\n");
pthread_mutex_unlock(&mutex);
printf("Thread unlocked mutex.\n");
return NULL;
}
int main() {
pthread_t thread;
pthread_create(&thread, NULL, thread_func, NULL);
printf("Main thread sleeping.\n");
sleep(1);
printf("Main thread waking up thread.\n");
pthread_mutex_lock(&mutex);
pthread_cond_signal(&condvar);
pthread_mutex_unlock(&mutex);
pthread_join(thread, NULL);
printf("Main thread exiting.\n");
return 0;
}
```
在这个例子中,主线程创建了一个新线程,并在新线程中调用 pthread_cond_wait 函数挂起任务。在主线程中,它会等待一秒钟,然后调用 pthread_cond_signal 函数来唤醒新线程。当新线程被唤醒后,它会重新获得 mutex,并从 pthread_cond_wait 函数返回。
相关推荐
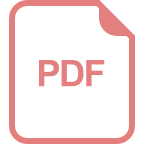
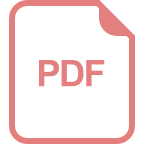
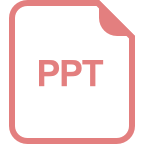














