linux dup命令
时间: 2023-10-08 19:11:55 浏览: 62
dup命令在Linux中是用于复制文件描述符的命令。
它的语法是:
```
int dup(int oldfd);
```
参数oldfd是需要复制的文件描述符,dup函数会返回一个新的文件描述符,这个新的文件描述符与oldfd指向的文件相同,但是它的文件描述符编号是当前未使用的最小编号。
dup函数的返回值是新的文件描述符,如果出错则返回-1。
dup函数的常见用法是将标准输入、标准输出或标准错误输出重定向到文件或管道中。例如:
```
int fd = open("file.txt", O_WRONLY|O_CREAT, 0644);
dup2(fd, STDOUT_FILENO); // 将标准输出重定向到文件
```
这里先打开一个文件,然后使用dup2函数将标准输出重定向到这个文件中。这样,所有输出到标准输出的内容都会被写入到文件中。
相关问题
linux用C语言实现shell命令<>重定向
在 Linux 中使用 C 语言实现 shell 命令 <> 的重定向,可以使用 dup2() 函数来实现。dup2() 函数可以将一个文件描述符的内容复制到另一个文件描述符中,从而实现文件重定向。
下面是一个简单的示例程序,可以实现 “<” 和 “>” 的文件重定向功能:
```c
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
int main(int argc, char *argv[]) {
int in_fd, out_fd;
char *in_file, *out_file, *cmd;
if (argc != 4) {
fprintf(stderr, "Usage: %s <input_file> <output_file> <command>\n", argv[0]);
return 1;
}
in_file = argv[1];
out_file = argv[2];
cmd = argv[3];
// 打开输入文件
in_fd = open(in_file, O_RDONLY);
if (in_fd == -1) {
perror("open input file");
return 2;
}
// 打开输出文件
out_fd = open(out_file, O_CREAT|O_WRONLY|O_TRUNC, 0644);
if (out_fd == -1) {
perror("open output file");
return 2;
}
// 将输入文件重定向到标准输入
if (dup2(in_fd, STDIN_FILENO) == -1) {
perror("dup2 input file");
return 3;
}
// 将输出文件重定向到标准输出
if (dup2(out_fd, STDOUT_FILENO) == -1) {
perror("dup2 output file");
return 3;
}
// 执行命令
if (system(cmd) == -1) {
perror("system command");
return 4;
}
// 关闭文件
close(in_fd);
close(out_fd);
return 0;
}
```
在上面的程序中,我们首先打开输入文件和输出文件,并将它们分别重定向到标准输入和标准输出。然后执行传入的命令,命令的输出将被重定向到输出文件中。最后关闭文件描述符。
使用该程序可以实现以下命令的效果:
```bash
$ ./redirect input.txt output.txt wc -l
```
该命令会将输入文件 input.txt 的内容通过 “<” 重定向到 wc 命令的标准输入中,将 wc 的输出通过 “>” 重定向到 output.txt 文件中。最后输出文件 output.txt 中行数的结果。
linux用C语言模拟shell命令并且实现重定向
要在 Linux 上使用 C 语言模拟 shell 命令,可以使用系统调用函数来执行命令。其中,`fork()` 函数用于创建子进程,`execvp()` 函数用于在子进程中执行命令。以下是一个简单的示例:
```c
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#include <sys/wait.h>
#include <fcntl.h>
#define MAX_ARGS 10
#define MAX_COMMAND_LENGTH 50
int main() {
char command[MAX_COMMAND_LENGTH];
char *args[MAX_ARGS];
int background = 0;
int redirect = 0;
char *redirect_file;
while (1) {
// 读取用户输入的命令
printf("myshell> ");
fgets(command, MAX_COMMAND_LENGTH, stdin);
command[strlen(command)-1] = '\0'; // 去掉换行符
// 解析命令参数
int i = 0;
args[i] = strtok(command, " ");
while (args[i] != NULL) {
i++;
args[i] = strtok(NULL, " ");
}
args[i] = NULL;
// 判断是否后台运行
if (args[i-1] != NULL && strcmp(args[i-1], "&") == 0) {
background = 1;
args[i-1] = NULL;
}
// 判断是否重定向输出
for (int j = 0; j < i; j++) {
if (strcmp(args[j], ">") == 0) {
redirect = 1;
args[j] = NULL;
redirect_file = args[j+1];
}
}
// 创建子进程执行命令
pid_t pid = fork();
if (pid == -1) {
perror("fork error");
exit(1);
} else if (pid == 0) {
// 子进程
if (redirect) {
int fd = open(redirect_file, O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (fd == -1) {
perror("open error");
exit(1);
}
dup2(fd, STDOUT_FILENO);
close(fd);
}
execvp(args[0], args);
perror("execvp error");
exit(1);
} else {
// 父进程
if (!background) {
waitpid(pid, NULL, 0);
}
}
}
return 0;
}
```
上述代码实现了一个简单的 shell,支持后台运行和重定向输出。例如,可以输入 `ls -l > output.txt` 命令将 `ls -l` 命令的输出重定向到 `output.txt` 文件中。
相关推荐
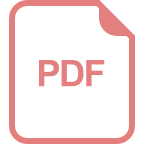
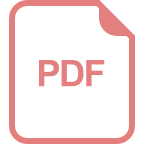
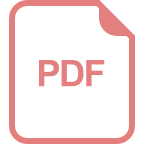












