Python开发背单词项目,实现四级英语词汇的中翻英、英翻中、背单词、记单词、背错词等功能。
时间: 2023-07-04 21:05:09 浏览: 210
好的,这个项目的实现可以分成几个步骤:
1. 数据准备:首先需要准备四级英语词汇的单词表,可以从网上下载或者自己整理。将单词表存储到本地文件中,可以使用csv或者txt格式。
2. 功能实现:根据需求,实现中翻英、英翻中、背单词、记单词、背错词等功能。
- 中翻英:输入中文单词,输出对应的英文单词。
- 英翻中:输入英文单词,输出对应的中文单词。
- 背单词:随机出题,提供中文或英文,用户输入对应的英文或中文。
- 记单词:逐一展示单词和其对应的翻译,让用户记忆。
- 背错词:展示用户上一次背诵时答错的单词,让用户重新背诵。
3. 用户界面:为了方便用户使用,可以使用tkinter或者PyQt等库实现一个简单的图形界面。
4. 优化:可以考虑加入单词读音、复习机制等功能,提高用户体验。
至于具体代码实现,这里提供一些思路供参考:
- 数据准备:将单词表存储到csv文件中,用pandas库读取csv文件并存储为字典形式,方便后续查询。
```python
import pandas as pd
df = pd.read_csv('word_list.csv', encoding='utf-8')
word_dict = df.set_index('中文').to_dict()['英文']
```
- 中翻英:
```python
def chinese_to_english(word_dict):
while True:
word = input('请输入中文单词:')
if word in word_dict:
print(word_dict[word])
else:
print('未找到该单词,请重新输入。')
```
- 英翻中:
```python
def english_to_chinese(word_dict):
while True:
word = input('请输入英文单词:')
for k, v in word_dict.items():
if v == word:
print(k)
break
else:
print('未找到该单词,请重新输入。')
```
- 背单词:
```python
import random
def recite_words(word_dict):
correct_count = 0
wrong_words = []
words = list(word_dict.keys())
while True:
word = random.choice(words)
direction = random.randint(0, 1)
if direction == 0:
ans = input(f"{word}的英文是:")
if ans == word_dict[word]:
correct_count += 1
print("回答正确!")
else:
wrong_words.append(word)
print(f"回答错误,正确答案是{word_dict[word]}。")
else:
ans = input(f"{word_dict[word]}的中文是:")
if ans == word:
correct_count += 1
print("回答正确!")
else:
wrong_words.append(word_dict[word])
print(f"回答错误,正确答案是{word}。")
if len(words) == 0:
print("恭喜你已经完成背单词!")
break
choice = input("是否继续?(y/n)")
if choice == 'n':
print(f"你一共答对了{correct_count}个单词。")
if wrong_words:
print("以下是你错过的单词:")
for wrong_word in wrong_words:
print(f"{wrong_word}: {word_dict[wrong_word]}")
break
```
- 记单词:
```python
def memorize_words(word_dict):
for k, v in word_dict.items():
print(f"{k}: {v}")
input("按任意键继续...")
```
- 背错词:
```python
def recite_wrong_words(wrong_words, word_dict):
correct_count = 0
while True:
if len(wrong_words) == 0:
print("你没有错过任何单词!")
break
word = random.choice(wrong_words)
direction = random.randint(0, 1)
if direction == 0:
ans = input(f"{word}的英文是:")
if ans == word_dict[word]:
correct_count += 1
print("回答正确!")
wrong_words.remove(word)
else:
print(f"回答错误,正确答案是{word_dict[word]}。")
else:
ans = input(f"{word_dict[word]}的中文是:")
if ans == word:
correct_count += 1
print("回答正确!")
wrong_words.remove(word)
else:
print(f"回答错误,正确答案是{word}。")
choice = input("是否继续?(y/n)")
if choice == 'n':
print(f"你一共答对了{correct_count}个单词。")
break
```
以上是一些简单的代码实现,可以根据实际需求进行修改和扩展。希望能对你有所帮助!
阅读全文
相关推荐
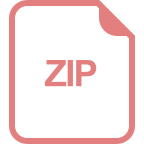
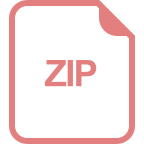
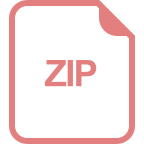














