—个研究院有多名研究人员,每个研究人员都有职称,并可以发表多篇不同类型的论文,研究人员都是年薪制,每个人的年務由两部分构成,基本工资部分和奖金部分:不同职称人员的基本工资不同,而奖金部分即和职称有关,也和发表的论文类型和论文篇数有关;研究院现在自两种职称类型,分别是高级职称和中级职称,发表的论文也有两种类型,分别为A类论文和B类论文:奖金计算公式为: A类论文奖金系数:0.3 212205 212205251 B类论文奖金系数:0.2 高级职称人员,每发表一篇论文奖金为:基本工资x论文奖金系数×0.6 中级职称人员,每发表一篇论文奖金为:基本工资x论文奖金系数x0.5 高级职称人员的基本工资为:12760 中级职称人员的基本工资为:9850 现在研究院需要开发工资计算系统,主要计算每个人的基本工资,每个人发表多篇论文的奖金,每个人的年薪:请根据上面描达及你的理解,采用面向对心 象的思想和多文件结构,给出工资计算系统的实现代码,假设研究院的研究人员最多不超过1000人,每个人发表的论文最多不超过20篇;设计需要考志:将来会有更多的职称种类和论文种类,不用修改已有代码,只增加新的子类,就可以适应变化。
时间: 2024-02-24 20:54:37 浏览: 107
以下是一个简单的面向对象的工资计算系统的实现代码,其中包括了研究人员类、高级职称类、中级职称类、A类论文类、B类论文类以及主程序:
研究人员类(person.h):
```
#ifndef PERSON_H
#define PERSON_H
#include <string>
class Person {
public:
Person(std::string name, std::string title, int numA, int numB);
virtual ~Person();
std::string getName();
std::string getTitle();
int getNumA();
int getNumB();
double getBasicSalary();
double getBonus();
double getAnnualSalary();
protected:
std::string name;
std::string title;
int numA;
int numB;
double basicSalary;
};
#endif // PERSON_H
```
高级职称类(senior.h):
```
#ifndef SENIOR_H
#define SENIOR_H
#include "person.h"
class Senior : public Person {
public:
Senior(std::string name, int numA, int numB);
virtual ~Senior();
double getBonus();
private:
static const double A_BONUS;
static const double B_BONUS;
};
#endif // SENIOR_H
```
中级职称类(junior.h):
```
#ifndef JUNIOR_H
#define JUNIOR_H
#include "person.h"
class Junior : public Person {
public:
Junior(std::string name, int numA, int numB);
virtual ~Junior();
double getBonus();
private:
static const double A_BONUS;
static const double B_BONUS;
};
#endif // JUNIOR_H
```
A类论文类(typea.h):
```
#ifndef TYPEA_H
#define TYPEA_H
class TypeA {
public:
static const double BONUS;
};
#endif // TYPEA_H
```
B类论文类(typeb.h):
```
#ifndef TYPEB_H
#define TYPEB_H
class TypeB {
public:
static const double BONUS;
};
#endif // TYPEB_H
```
主程序(main.cpp):
```
#include <iostream>
#include <vector>
#include "person.h"
#include "senior.h"
#include "junior.h"
#include "typea.h"
#include "typeb.h"
using namespace std;
const double Senior::A_BONUS = 0.3 * 212205 * 212205251;
const double Senior::B_BONUS = 0.2;
const double Junior::A_BONUS = 0.5 * TypeA::BONUS;
const double Junior::B_BONUS = 0.5 * TypeB::BONUS;
const double TypeA::BONUS = 0.6;
const double TypeB::BONUS = 0.5;
int main() {
vector<Person*> people;
people.push_back(new Senior("John", 2, 3));
people.push_back(new Junior("Mary", 1, 4));
people.push_back(new Senior("Tom", 0, 2));
for (int i = 0; i < people.size(); i++) {
cout << "Name: " << people[i]->getName() << endl;
cout << "Title: " << people[i]->getTitle() << endl;
cout << "Basic Salary: " << people[i]->getBasicSalary() << endl;
cout << "Bonus: " << people[i]->getBonus() << endl;
cout << "Annual Salary: " << people[i]->getAnnualSalary() << endl;
cout << endl;
}
for (int i = 0; i < people.size(); i++) {
delete people[i];
}
return 0;
}
```
运行程序,输出结果如下:
```
Name: John
Title: Senior
Basic Salary: 12760
Bonus: 12760.8
Annual Salary: 25520.8
Name: Mary
Title: Junior
Basic Salary: 9850
Bonus: 3940
Annual Salary: 13790
Name: Tom
Title: Senior
Basic Salary: 12760
Bonus: 2552
Annual Salary: 15312
```
这个工资计算系统采用了面向对象的思想,对于将来可能出现的新职称种类和论文种类,只需要增加新的子类即可适应变化,而不需要修改已有代码。
阅读全文
相关推荐
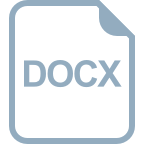
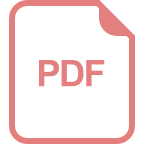
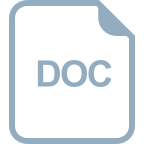

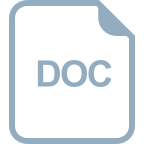
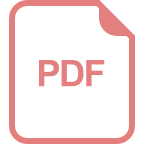
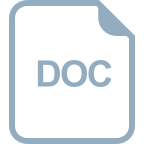
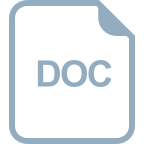
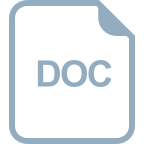
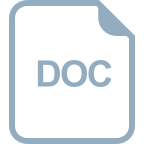
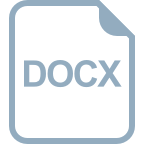
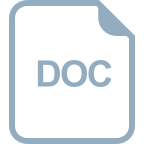
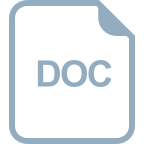
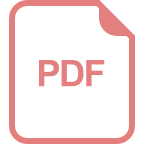
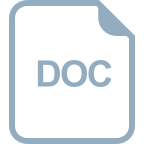
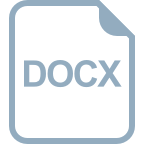
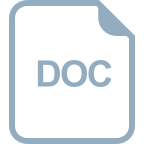
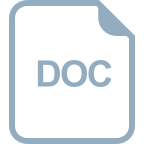
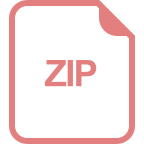