qt蓝牙串口调试助手代码
时间: 2023-06-27 19:07:39 浏览: 449
以下是一个简单的 Qt 蓝牙串口调试助手的示例代码:
```cpp
#include <QCoreApplication>
#include <QBluetoothDeviceDiscoveryAgent>
#include <QBluetoothSocket>
#include <QSerialPort>
#include <QSerialPortInfo>
#include <QTimer>
class BluetoothSerialPort : public QObject {
Q_OBJECT
public:
BluetoothSerialPort(QObject *parent = nullptr)
: QObject(parent)
, m_socket(new QBluetoothSocket(QBluetoothServiceInfo::RfcommProtocol, this))
{
connect(m_socket, &QBluetoothSocket::connected, this, &BluetoothSerialPort::connected);
connect(m_socket, &QBluetoothSocket::disconnected, this, &BluetoothSerialPort::disconnected);
connect(m_socket, &QBluetoothSocket::readyRead, this, &BluetoothSerialPort::readyRead);
connect(m_socket, QOverload<QBluetoothSocket::SocketError>::of(&QBluetoothSocket::error), this, &BluetoothSerialPort::error);
}
public slots:
void connectToDevice(const QString &address)
{
m_socket->connectToService(QBluetoothAddress(address), QBluetoothUuid(QBluetoothUuid::SerialPort));
}
void disconnect()
{
m_socket->disconnectFromService();
}
void write(const QByteArray &data)
{
m_socket->write(data);
}
signals:
void connected();
void disconnected();
void readyRead(const QByteArray &data);
void error(const QString &message);
private:
QBluetoothSocket *m_socket;
};
class SerialPort : public QObject {
Q_OBJECT
public:
SerialPort(QObject *parent = nullptr)
: QObject(parent)
, m_serialPort(new QSerialPort(this))
{
connect(m_serialPort, &QSerialPort::readyRead, this, &SerialPort::readyRead);
connect(m_serialPort, &QSerialPort::errorOccurred, this, &SerialPort::error);
}
public slots:
void open(const QString &portName, int baudRate)
{
m_serialPort->setPortName(portName);
m_serialPort->setBaudRate(baudRate);
m_serialPort->setDataBits(QSerialPort::Data8);
m_serialPort->setParity(QSerialPort::NoParity);
m_serialPort->setStopBits(QSerialPort::OneStop);
m_serialPort->setFlowControl(QSerialPort::NoFlowControl);
if (m_serialPort->open(QIODevice::ReadWrite)) {
emit opened();
} else {
emit error(tr("Failed to open serial port: %1").arg(m_serialPort->errorString()));
}
}
void close()
{
m_serialPort->close();
emit closed();
}
void write(const QByteArray &data)
{
m_serialPort->write(data);
}
signals:
void opened();
void closed();
void readyRead(const QByteArray &data);
void error(const QString &message);
private:
QSerialPort *m_serialPort;
};
class BluetoothSerialPortDiscovery : public QObject {
Q_OBJECT
public:
BluetoothSerialPortDiscovery(QObject *parent = nullptr)
: QObject(parent)
, m_agent(new QBluetoothDeviceDiscoveryAgent(this))
{
connect(m_agent, &QBluetoothDeviceDiscoveryAgent::deviceDiscovered, this, &BluetoothSerialPortDiscovery::deviceDiscovered);
connect(m_agent, &QBluetoothDeviceDiscoveryAgent::finished, this, &BluetoothSerialPortDiscovery::finished);
}
public slots:
void start()
{
m_devices.clear();
m_agent->start();
}
signals:
void deviceDiscovered(const QString &name, const QString &address);
void finished();
private:
QBluetoothDeviceDiscoveryAgent *m_agent;
QList<QBluetoothDeviceInfo> m_devices;
private slots:
void deviceDiscovered(const QBluetoothDeviceInfo &device)
{
if (device.coreConfigurations() & QBluetoothDeviceInfo::SerialPort) {
m_devices.append(device);
emit this->deviceDiscovered(device.name(), device.address().toString());
}
}
void finished()
{
emit this->finished();
}
};
class BluetoothSerialPortAssist : public QObject {
Q_OBJECT
public:
BluetoothSerialPortAssist(QObject *parent = nullptr)
: QObject(parent)
, m_serialPort(new SerialPort(this))
, m_bluetoothSerialPort(new BluetoothSerialPort(this))
, m_discovery(new BluetoothSerialPortDiscovery(this))
{
connect(m_discovery, &BluetoothSerialPortDiscovery::deviceDiscovered, this, &BluetoothSerialPortAssist::deviceDiscovered);
connect(m_serialPort, &SerialPort::readyRead, this, &BluetoothSerialPortAssist::serialPortReadyRead);
connect(m_serialPort, &SerialPort::error, this, &BluetoothSerialPortAssist::serialPortError);
connect(m_bluetoothSerialPort, &BluetoothSerialPort::connected, this, &BluetoothSerialPortAssist::bluetoothSerialPortConnected);
connect(m_bluetoothSerialPort, &BluetoothSerialPort::disconnected, this, &BluetoothSerialPortAssist::bluetoothSerialPortDisconnected);
connect(m_bluetoothSerialPort, &BluetoothSerialPort::readyRead, this, &BluetoothSerialPortAssist::bluetoothSerialPortReadyRead);
connect(m_bluetoothSerialPort, &BluetoothSerialPort::error, this, &BluetoothSerialPortAssist::bluetoothSerialPortError);
}
public slots:
void startBluetoothDiscovery()
{
m_discovery->start();
}
void connectToBluetoothDevice(const QString &address)
{
m_bluetoothSerialPort->connectToDevice(address);
}
void disconnectFromBluetoothDevice()
{
m_bluetoothSerialPort->disconnect();
}
void openSerialPort(const QString &portName, int baudRate)
{
m_serialPort->open(portName, baudRate);
}
void closeSerialPort()
{
m_serialPort->close();
}
void writeData(const QByteArray &data)
{
if (m_bluetoothSerialPort->state() == QBluetoothSocket::ConnectedState) {
m_bluetoothSerialPort->write(data);
} else if (m_serialPort->isOpen()) {
m_serialPort->write(data);
}
}
signals:
void bluetoothDeviceDiscovered(const QString &name, const QString &address);
void bluetoothSerialPortConnected();
void bluetoothSerialPortDisconnected();
void bluetoothSerialPortReadyRead(const QByteArray &data);
void bluetoothSerialPortError(const QString &message);
void serialPortOpened();
void serialPortClosed();
void serialPortReadyRead(const QByteArray &data);
void serialPortError(const QString &message);
private:
SerialPort *m_serialPort;
BluetoothSerialPort *m_bluetoothSerialPort;
BluetoothSerialPortDiscovery *m_discovery;
private slots:
void deviceDiscovered(const QString &name, const QString &address)
{
emit this->bluetoothDeviceDiscovered(name, address);
}
void serialPortReadyRead(const QByteArray &data)
{
emit this->serialPortReadyRead(data);
}
void serialPortError(const QString &message)
{
emit this->serialPortError(message);
}
void bluetoothSerialPortConnected()
{
emit this->bluetoothSerialPortConnected();
}
void bluetoothSerialPortDisconnected()
{
emit this->bluetoothSerialPortDisconnected();
}
void bluetoothSerialPortReadyRead(const QByteArray &data)
{
emit this->bluetoothSerialPortReadyRead(data);
}
void bluetoothSerialPortError(const QString &message)
{
emit this->bluetoothSerialPortError(message);
}
};
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
BluetoothSerialPortAssist assist;
QObject::connect(&assist, &BluetoothSerialPortAssist::bluetoothDeviceDiscovered, [](const QString &name, const QString &address) {
qDebug() << "Bluetooth device discovered:" << name << address;
});
QObject::connect(&assist, &BluetoothSerialPortAssist::bluetoothSerialPortConnected, []() {
qDebug() << "Bluetooth serial port connected";
});
QObject::connect(&assist, &BluetoothSerialPortAssist::bluetoothSerialPortDisconnected, []() {
qDebug() << "Bluetooth serial port disconnected";
});
QObject::connect(&assist, &BluetoothSerialPortAssist::bluetoothSerialPortReadyRead, [](const QByteArray &data) {
qDebug() << "Bluetooth serial port data received:" << data;
});
QObject::connect(&assist, &BluetoothSerialPortAssist::bluetoothSerialPortError, [](const QString &message) {
qDebug() << "Bluetooth serial port error:" << message;
});
QObject::connect(&assist, &BluetoothSerialPortAssist::serialPortOpened, []() {
qDebug() << "Serial port opened";
});
QObject::connect(&assist, &BluetoothSerialPortAssist::serialPortClosed, []() {
qDebug() << "Serial port closed";
});
QObject::connect(&assist, &BluetoothSerialPortAssist::serialPortReadyRead, [](const QByteArray &data) {
qDebug() << "Serial port data received:" << data;
});
QObject::connect(&assist, &BluetoothSerialPortAssist::serialPortError, [](const QString &message) {
qDebug() << "Serial port error:" << message;
});
assist.startBluetoothDiscovery();
QTimer::singleShot(5000, [&assist]() {
assist.connectToBluetoothDevice("00:11:22:33:44:55");
assist.openSerialPort("/dev/ttyUSB0", 9600);
assist.writeData("Hello, world!");
});
QTimer::singleShot(10000, [&assist]() {
assist.disconnectFromBluetoothDevice();
assist.closeSerialPort();
});
return app.exec();
}
#include "main.moc"
```
此示例程序包括以下功能:
- 使用 `QBluetoothDeviceDiscoveryAgent` 搜索蓝牙串口设备并展示设备名称和 MAC 地址;
- 使用 `QBluetoothSocket` 连接蓝牙串口设备;
- 使用 `QSerialPort` 连接本地串口设备;
- 从蓝牙串口设备或本地串口设备读取数据;
- 将数据写入蓝牙串口设备或本地串口设备。
在使用此代码之前,请确保已经安装了 Qt Bluetooth 和 Qt SerialPort 模块。
阅读全文
相关推荐
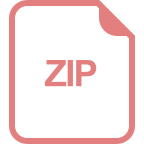
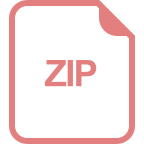














