如何设置静态数组python
时间: 2024-03-19 16:44:25 浏览: 6
在Python中,数组被称为列表(List),列表是一种动态数据结构,可以随意添加、删除和修改元素。如果要创建一个静态数组,也就是在创建列表时确定长度,可以使用以下方式:
```python
# 定义一个长度为5的静态数组
arr = [0] * 5
# 或者使用以下方式
arr = [None] * 5
# 将静态数组的第1个元素赋值为1
arr[0] = 1
# 输出静态数组
print(arr) # 输出 [1, 0, 0, 0, 0]
```
在上面的代码中,我们使用`[0] * 5`或`[None] * 5`的方式创建一个长度为5的静态数组。由于Python中的列表是动态数组,因此我们可以在创建后随意修改其中的元素。
相关问题
Python集合数组
Python中的集合和数组有一些不同的特点和用途。
集合(Set)是一种无序且不重复的数据结构,它由一组元素组成,可以进行基本的集合操作,如并集、交集、差集等。在Python中,可以使用set()函数或者使用大括号{}来创建一个集合。例如:
```python
my_set = set([1, 2, 3, 3, 4, 5]) # 使用set()函数创建集合
my_set2 = {4, 5, 6, 7} # 使用大括号创建集合
```
数组(Array)是一种有序的数据结构,它由一组相同类型的元素按照一定顺序排列而成。在Python中,可以使用列表(List)来模拟数组的功能。列表可以包含不同类型的元素,并且可以进行增删改查等操作。例如:
```python
my_array = [1, 2, 3, 4, 5] # 创建一个列表作为数组
```
需要注意的是,Python中的列表是动态的,可以根据需要动态调整大小,而且可以包含不同类型的元素。因此,与其他编程语言中的静态数组相比,Python中的列表更加灵活和方便使用。
希望这个回答对你有所帮助!如果你有其他问题,请随时提问。
onnx 模型静态输入 python
在Python中,可以使用ONNX模型来进行静态输入。首先,需要安装ONNX库,并确保已经有训练好的ONNX模型文件。然后,可以使用ONNX库中的函数将模型加载到Python中,然后通过静态输入的方式向模型传入数据进行推理。
首先,需要导入ONNX库并加载模型文件。接下来,需要准备输入数据,可以是一个数组或者一个张量,根据模型的输入要求进行相应的数据类型和格式处理。然后,调用模型的推理函数,将准备好的输入数据传入模型中进行推理,并获取模型输出的结果。
需要注意的是,在使用静态输入的过程中,输入数据的格式和类型需要和模型的输入要求相匹配,以确保能够顺利地进行推理。另外,可以根据需求对模型输出的结果进行处理和后续的应用,比如进行分类、检测、分割等任务。
总之,在Python中,可以通过ONNX库来实现对ONNX模型的静态输入,通过合适的数据准备和模型推理,可以方便地使用训练好的ONNX模型进行预测和推理,满足不同的应用需求。
相关推荐
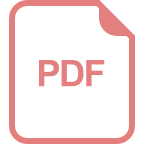
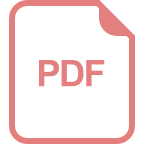












