代码改错#include <iostream> #include <string.h> #include <stdio.h> using namespace std; class String { public: String() {} String(char str[20]); char Str[20]; friend istream& operator>>(istream& in, String& s); friend ostream& operator<<(ostream& out, String& s); }; String::String(char str[20]) { size_t len = strlen(str); strcpy_s(Str,len, str); } istream& operator>>(istream& in, String& s) { char p[20]; in.getline(p, 20); size_t len = strlen(p); strcpy_s(s.Str,len, p); return in; } ostream& operator<<(ostream& out, String& s) { out << s.Str; return out; } template<class TNo, class TScore, int num>//TNo和TScore为参数化类型 class Student { private: TNo StudentID; //参数化类型,存储姓名 TScore score[num]; //参数化类型数组,存储num门课程的分数 public: void Input();//数据的录入 TScore MaxScore(); //查找score的最大值并返回该值 void Update(TScore sscore, int i);//更新学生的第i门课程成绩为sscore void SelectSort(); //采用选择排序法对学生成绩进行升序排列 void Print(); //输出所有学生的信息 }; template<class TNo, class TScore, int num> void Student<TNo,TScore,num>::Input() { cin >> StudentID; for (int i = 0; i < 3; i++) { cin >> score[i]; } } template<class TNo, class TScore, int num> TScore Student<TNo, TScore, num>::MaxScore() { TScore x = 0; if (num != 0) { for (int i = 0; i < num; i++) { if (score[i] > x) { x = score[i]; } } } return x; } template<class TNo, class TScore, int num> void Student<TNo, TScore, num>::Update(TScore sscore, int i) { if (i >= 0 && i < num) { score[i] = sscore; } } template<class TNo, class TScore, int num> void Student<TNo, TScore, num>::SelectSort() { for (int i = 0; i < num; i++) { TScore a = score[i]; for (int j = i; i < num; j++) { if (score[i] < a) { a = score[i]; } } score[i] = a; } } template<class TNo, class TScore, int num> void Student<TNo, TScore, num>::Print() { cout << MaxScore() << endl; cout << StudentID << "\t"; cout << score; } int main(void) { Student <String, float, 3> s; s.Input(); s.SelectSort(); s.Print(); return 0; }
时间: 2024-01-21 18:02:20 浏览: 29
这段代码有几处错误:
1. 在`String`类的构造函数中,应该使用`strcpy`函数而不是`strcpy_s`函数,因为`strcpy_s`函数是C11标准新增的函数,不是所有编译器都支持。同时,`strcpy_s`函数的第二个参数应该是字符数组的长度,而不是字符串的长度。
2. 在`operator<<`函数中,输出字符串时应该使用`s.Str`而不是`s`。
3. 在`SelectSort`函数中,内层循环的判断条件应该是`j < num`而不是`i < num`。
4. 在`Print`函数中,不能直接输出`score`数组,需要对每个成绩使用循环进行输出。
下面是修改后的代码:
```c++
#include <iostream>
#include <string.h>
#include <stdio.h>
using namespace std;
class String {
public:
String() {}
String(char str[20]);
char Str[20];
friend istream& operator>>(istream& in, String& s);
friend ostream& operator<<(ostream& out, String& s);
};
String::String(char str[20]) {
size_t len = strlen(str);
strcpy(Str, str);
}
istream& operator>>(istream& in, String& s) {
char p[20];
in.getline(p, 20);
size_t len = strlen(p);
strcpy(s.Str, p);
return in;
}
ostream& operator<<(ostream& out, String& s) {
out << s.Str;
return out;
}
template<class TNo, class TScore, int num>
class Student {
private:
TNo StudentID;
TScore score[num];
public:
void Input();
TScore MaxScore();
void Update(TScore sscore, int i);
void SelectSort();
void Print();
};
template<class TNo, class TScore, int num>
void Student<TNo, TScore, num>::Input() {
cin >> StudentID;
for (int i = 0; i < num; i++) {
cin >> score[i];
}
}
template<class TNo, class TScore, int num>
TScore Student<TNo, TScore, num>::MaxScore() {
TScore x = 0;
if (num != 0) {
for (int i = 0; i < num; i++) {
if (score[i] > x) {
x = score[i];
}
}
}
return x;
}
template<class TNo, class TScore, int num>
void Student<TNo, TScore, num>::Update(TScore sscore, int i) {
if (i >= 0 && i < num) {
score[i] = sscore;
}
}
template<class TNo, class TScore, int num>
void Student<TNo, TScore, num>::SelectSort() {
for (int i = 0; i < num; i++) {
TScore a = score[i];
for (int j = i; j < num; j++) {
if (score[j] < a) {
a = score[j];
}
}
score[i] = a;
}
}
template<class TNo, class TScore, int num>
void Student<TNo, TScore, num>::Print() {
cout << MaxScore() << endl;
cout << StudentID << "\t";
for (int i = 0; i < num; i++) {
cout << score[i] << "\t";
}
}
int main(void) {
Student<String, float, 3> s;
s.Input();
s.SelectSort();
s.Print();
return 0;
}
```
相关推荐
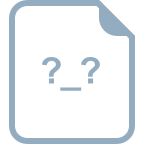















