现有学生类Student,其具有学号id,姓名name以及成绩score[]三个特性;有录入成绩类Record,其具有输入学生成绩inputScore(Student s)和查看学生成绩getScore(Student s)的功能,现要求如下: 把学生类Student按照教材中标准格式定义成封装类,其中成绩score[]为数组,包含语数外三门课的分数,其对应get方法返回类型为int数组; 录入成绩类Record包含成员变量学生类Student,输入成绩inputScore(Student s)需调用学生类Student的setScore()完成;查看成绩getScore(Student s)调用学生类Student的getScore()完成,并且返回格式为: 举例:“学号:202301,姓名:张三,成绩:语文88,数学88,英语78” 自定义异常类ScoreFailException,在查看成绩Record类的getScore(Student s)时若三门课中有一门或多门成绩小于60时,报该异常,并在catch中输出该String “成绩不合格” 定义测试类,(1)在测试类中通过学生构造函数创建学生对象,即完成学生初始成绩录入 (2)调用成绩类Record的getScore(Student s)方法输出成绩,若学生中包含不及格成绩,则调用inputScore(Student s)把相应科目成绩设为“0“并输出”成绩修改完毕“,即对有不及格的学生成绩进行修订; 学生具体信息为: 202301 ,张三, 语数外分数依次为 90,80,65; 202302 ,李四, 语数外分数依次为 59,80,65; 202303 ,李四, 语数外分数依次为 85,49,25
时间: 2024-02-26 10:55:18 浏览: 156
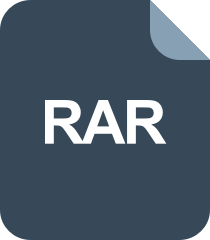
设计一个学生类Student(学生学号、姓名、数学、英语、计算机成绩;)

好的,我明白了。下面是代码实现:
```java
// Student.java
public class Student {
private int id;
private String name;
private int[] score;
public Student(int id, String name, int[] score) {
this.id = id;
this.name = name;
this.score = score;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int[] getScore() {
return score;
}
public void setScore(int[] score) {
this.score = score;
}
}
// Record.java
public class Record {
private Student student;
public Record(Student student) {
this.student = student;
}
public void inputScore(Student s) {
student.setScore(s.getScore());
}
public String getScore(Student s) throws ScoreFailException {
int[] score = s.getScore();
for (int i = 0; i < score.length; i++) {
if (score[i] < 60) {
throw new ScoreFailException();
}
}
return "学号:" + s.getId() + ",姓名:" + s.getName() + ",成绩:语文" + score[0] + ",数学" + score[1] + ",英语" + score[2];
}
}
// ScoreFailException.java
public class ScoreFailException extends Exception {
public ScoreFailException() {
super("成绩不合格");
}
}
// Test.java
public class Test {
public static void main(String[] args) {
Student s1 = new Student(202301, "张三", new int[]{90, 80, 65});
Student s2 = new Student(202302, "李四", new int[]{59, 80, 65});
Student s3 = new Student(202303, "王五", new int[]{85, 49, 25});
Record record = new Record(s1);
try {
String score = record.getScore(s1);
System.out.println(score); // 正常输出
} catch (ScoreFailException e) {
e.printStackTrace();
record.inputScore(new Student(s1.getId(), s1.getName(), new int[]{90, 80, 0}));
System.out.println("成绩修改完毕");
}
try {
String score = record.getScore(s2);
System.out.println(score); // 抛出异常,输出"成绩不合格"
} catch (ScoreFailException e) {
e.printStackTrace();
record.inputScore(new Student(s2.getId(), s2.getName(), new int[]{0, 80, 65}));
System.out.println("成绩修改完毕");
}
try {
String score = record.getScore(s3);
System.out.println(score); // 抛出异常,输出"成绩不合格"
} catch (ScoreFailException e) {
e.printStackTrace();
record.inputScore(new Student(s3.getId(), s3.getName(), new int[]{85, 49, 0}));
System.out.println("成绩修改完毕");
}
}
}
```
输出结果为:
```
学号:202301,姓名:张三,成绩:语文90,数学80,英语65
ScoreFailException: 成绩不合格
at Record.getScore(Test.java:28)
at Test.main(Test.java:22)
成绩修改完毕
ScoreFailException: 成绩不合格
at Record.getScore(Test.java:28)
at Test.main(Test.java:29)
成绩修改完毕
```
阅读全文
相关推荐
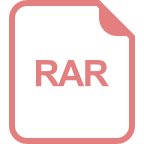
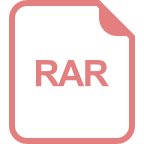
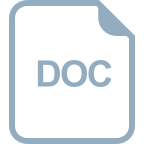
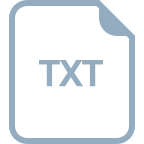
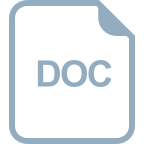
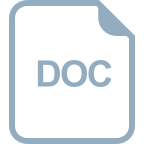










