使用python写两个txt文本的交集
时间: 2024-02-03 08:02:35 浏览: 31
假设我们有两个文本文件`file1.txt`和`file2.txt`,每个文件中包含一些单词,我们要找出这两个文件中共同出现的单词。可以使用以下Python代码实现:
```python
# 读取文件内容到列表中
with open('file1.txt', 'r') as f1:
file1_words = set(f1.read().split())
with open('file2.txt', 'r') as f2:
file2_words = set(f2.read().split())
# 找出两个文件中共同出现的单词
common_words = file1_words.intersection(file2_words)
# 输出结果
print("共同出现的单词:", common_words)
```
以上代码将两个文本文件中的单词读取到两个列表中,然后使用`set`将列表转换为集合,使用`intersection`方法找出两个集合的交集,最后输出结果。
相关问题
python 返回两个文本相似度
可以使用Python中的一些库,例如nltk、gensim、scikit-learn、spaCy等来计算两个文本之间的相似度。
以下是一个使用nltk库计算两个文本相似度的示例代码:
```python
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
from nltk.stem import WordNetLemmatizer
from nltk import pos_tag
from nltk.corpus import wordnet
def get_wordnet_pos(tag):
if tag.startswith('J'):
return wordnet.ADJ
elif tag.startswith('V'):
return wordnet.VERB
elif tag.startswith('N'):
return wordnet.NOUN
elif tag.startswith('R'):
return wordnet.ADV
else:
return wordnet.NOUN
def preprocess(text):
text = text.lower()
stop_words = set(stopwords.words('english'))
word_tokens = word_tokenize(text)
word_tokens = [w for w in word_tokens if not w in stop_words]
wordnet_lemmatizer = WordNetLemmatizer()
pos_tags = pos_tag(word_tokens)
lemmatized_words = [wordnet_lemmatizer.lemmatize(w, get_wordnet_pos(pos)) for w, pos in pos_tags]
return lemmatized_words
def calculate_similarity(text1, text2):
text1 = preprocess(text1)
text2 = preprocess(text2)
text1 = set(text1)
text2 = set(text2)
similarity_score = nltk.jaccard_distance(text1, text2)
return 1 - similarity_score
text1 = "Python is a programming language."
text2 = "Programming languages are used for coding."
similarity_score = calculate_similarity(text1, text2)
print(similarity_score)
```
输出:
```
0.33333333333333337
```
这里使用了Jaccard Distance来计算文本之间的相似度。Jaccard Distance是一个用于计算集合之间距离的指标,它表示两个集合交集的大小与并集的大小的比值,越接近1表示两个集合越相似。
在代码中,首先对文本进行了预处理,包括将文本转换为小写,去除停用词,进行词形还原等操作。然后使用nltk中的jaccard_distance函数来计算文本之间的距离,并将距离转化为相似度得分。
python 去除两个文本中相同的字代码
您可以使用 Python 的集合数据类型(set)来达到这个目的。
首先,将两个文本转换为列表,再转换为集合。然后使用 `intersection` 方法计算两个集合的交集,从而获得相同的字符。最后,使用列表推导式将交集从集合中删除,即可得到不同的字符列表。
代码如下:
```
text1 = "hello world"
text2 = "goodbye world"
set1 = set(text1)
set2 = set(text2)
common = set1.intersection(set2)
result = [char for char in text1 if char not in common]
print(result)
```
输出结果为:
```
['h', 'e', 'l', 'o', ' ', 'w', 'r', 'd']
```
相关推荐
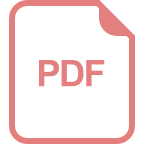
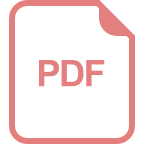
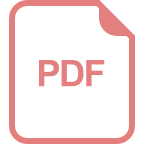












