多个BufferedInputStream流和多个BufferedOutputStream流合并成一个压缩流返回给前端一个zip文件
时间: 2024-03-24 11:13:56 浏览: 13
可以使用Java的ZipOutputStream类来实现将多个文件压缩为一个zip文件并返回给前端。具体实现步骤如下:
1. 创建一个空的zip文件,并用ZipOutputStream类将其包装起来。
```java
ZipOutputStream zipOut = new ZipOutputStream(new FileOutputStream("example.zip"));
```
2. 创建多个BufferedInputStream流,分别读取需要压缩的文件。
```java
BufferedInputStream in1 = new BufferedInputStream(new FileInputStream("file1.txt"));
BufferedInputStream in2 = new BufferedInputStream(new FileInputStream("file2.txt"));
```
3. 创建多个ZipEntry对象,分别为每个需要压缩的文件创建一个ZipEntry对象,并将其添加到ZipOutputStream中。
```java
zipOut.putNextEntry(new ZipEntry("file1.txt"));
byte[] bytes = new byte[1024];
int length;
while ((length = in1.read(bytes)) >= 0) {
zipOut.write(bytes, 0, length);
}
in1.close();
zipOut.closeEntry();
zipOut.putNextEntry(new ZipEntry("file2.txt"));
while ((length = in2.read(bytes)) >= 0) {
zipOut.write(bytes, 0, length);
}
in2.close();
zipOut.closeEntry();
```
4. 关闭ZipOutputStream流。
```java
zipOut.close();
```
完整的代码示例:
```java
public static void compressFilesToZip(List<File> files, HttpServletResponse response) throws Exception {
// 设置response的Header
response.setContentType("application/zip");
response.setHeader("Content-Disposition", "attachment;filename=example.zip");
// 创建一个空的zip文件,并用ZipOutputStream类将其包装起来
ZipOutputStream zipOut = new ZipOutputStream(response.getOutputStream());
for (File file : files) {
// 创建BufferedInputStream流,读取需要压缩的文件
BufferedInputStream in = new BufferedInputStream(new FileInputStream(file));
// 为每个需要压缩的文件创建一个ZipEntry对象,并将其添加到ZipOutputStream中
zipOut.putNextEntry(new ZipEntry(file.getName()));
byte[] bytes = new byte[1024];
int length;
while ((length = in.read(bytes)) >= 0) {
zipOut.write(bytes, 0, length);
}
in.close();
zipOut.closeEntry();
}
// 关闭ZipOutputStream流
zipOut.close();
}
```
相关推荐
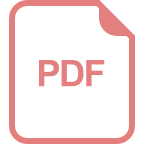
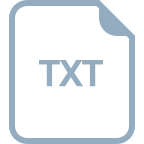
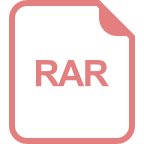














