八皇后问题,是一个古老而著名的问题,是回溯算法的典型案例。该问题是国际西洋棋棋手马克斯·贝瑟尔于 1848 年 提出:在 8×8 格的国际象棋上摆放八个皇后,使其不能互相攻击,即:任意两个皇后都不能处于同一行、同一列或同 一斜线上,问有多少种摆法,用Java算法解决
时间: 2023-11-27 11:03:56 浏览: 179
以下是用Java实现八皇后问题的代码:
```java
public class EightQueens {
private static final int BOARD_SIZE = 8;
private int[] queens;
public EightQueens() {
queens = new int[BOARD_SIZE];
}
public void solve() {
placeQueen(0);
}
private void placeQueen(int row) {
if (row == BOARD_SIZE) {
printQueens();
return;
}
for (int col = 0; col < BOARD_SIZE; col++) {
if (isValid(row, col)) {
queens[row] = col;
placeQueen(row + 1);
}
}
}
private boolean isValid(int row, int col) {
for (int i = 0; i < row; i++) {
int otherCol = queens[i];
if (col == otherCol) {
return false;
}
int colDiff = Math.abs(col - otherCol);
int rowDiff = row - i;
if (colDiff == rowDiff) {
return false;
}
}
return true;
}
private void printQueens() {
System.out.println("=======================");
for (int row = 0; row < BOARD_SIZE; row++) {
for (int col = 0; col < BOARD_SIZE; col++) {
if (queens[row] == col) {
System.out.print("Q ");
} else {
System.out.print(". ");
}
}
System.out.println();
}
System.out.println("=======================");
}
public static void main(String[] args) {
EightQueens solver = new EightQueens();
solver.solve();
}
}
```
在这个实现中,我们使用了一个一维数组来表示每一行皇后的位置,数组的下标表示行号,数组的值表示列号。在递归过程中,我们依次尝试在每一行放置皇后,如果当前位置合法,则继续尝试下一行;如果当前位置不合法,则回溯到上一行重新尝试。在回溯算法的框架下,我们可以很容易地实现八皇后问题的求解。
阅读全文
相关推荐
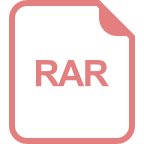
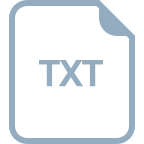

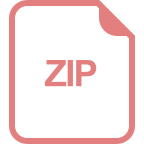
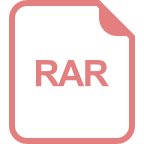
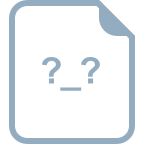
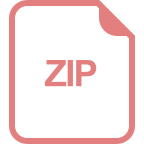
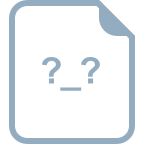
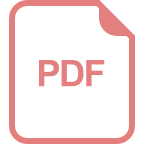
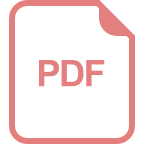
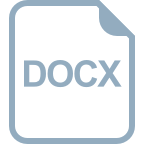
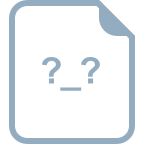
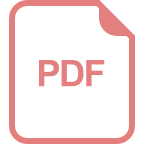
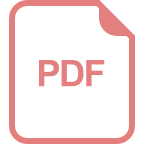
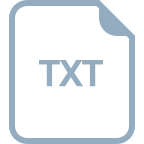
