基于蚁群算法解决物流配送路径,利用分区配送使路径最优,生成坐标图路径,Python代码
时间: 2024-09-08 20:01:19 浏览: 53
蚁群算法是一种模拟自然界蚂蚁觅食行为的优化算法,它通过模拟蚂蚁寻找食物过程中释放信息素来找到最短路径的行为。在物流配送路径优化问题中,蚁群算法可以用来寻找一条或多条最短或成本最低的配送路径。分区配送是将配送区域划分为若干个小区域,每个区域由一个配送点负责,这样可以减少单个配送点的配送距离和时间,提高配送效率。
以下是使用蚁群算法来解决物流配送路径问题的大致思路,并附上一个简化的Python代码示例:
1. 初始化参数:包括蚁群的数量、信息素的重要程度、启发式因子的重要程度、信息素的挥发系数、迭代次数等。
2. 构造初始信息素矩阵:基于坐标图上的点,初始化每个点间的信息素浓度。
3. 蚂蚁构造解:每只蚂蚁根据信息素浓度和启发式因子(如距离倒数)来选择下一个节点,直到完成一条路径。
4. 更新信息素:每次迭代后,根据蚂蚁走过的路径更新信息素,信息素浓度高的路径被更多蚂蚁选择,进而得到加强。
5. 重复步骤3和4,直到满足迭代次数或其他停止条件。
6. 输出最优路径。
以下是一个简化的Python代码示例,用于说明如何使用蚁群算法来优化路径:
```python
import numpy as np
# 假设有一个坐标图,需要找到最短路径
coordinates = np.array([[1, 2], [2, 5], [3, 3], [4, 1], [5, 4]]) # 示例坐标点
# 蚁群算法参数设置
num_ants = 10 # 蚂蚁数量
num_iterations = 50 # 迭代次数
decay = 0.5 # 信息素挥发系数
alpha = 1 # 信息素重要程度
beta = 2 # 启发式因子重要程度
# 初始化信息素矩阵
pheromone = np.ones((len(coordinates), len(coordinates)))
# 计算距离矩阵
def calculate_distance_matrix(coords):
dist_matrix = np.zeros((len(coords), len(coords)))
for i in range(len(coords)):
for j in range(len(coords)):
dist_matrix[i][j] = np.linalg.norm(coords[i] - coords[j])
return dist_matrix
dist_matrix = calculate_distance_matrix(coordinates)
# 蚁群算法主循环
for iteration in range(num_iterations):
all_paths = []
all_path_lengths = []
for ant in range(num_ants):
# 随机选择起始点
start = np.random.randint(len(coordinates))
path = [start]
unvisited = list(range(len(coordinates)))
unvisited.remove(start)
while unvisited:
current = path[-1]
# 计算转移概率
transition_prob = np.zeros(len(unvisited))
for i, next in enumerate(unvisited):
pheromone_value = pheromone[current][next]**alpha
heuristic_value = (1 / dist_matrix[current][next])**beta
transition_prob[i] = pheromone_value * heuristic_value
# 轮盘赌选择下一个节点
transition_prob /= np.sum(transition_prob)
next = np.random.choice(unvisited, p=transition_prob)
path.append(next)
unvisited.remove(next)
# 计算路径长度并记录
path_length = sum([dist_matrix[path[i]][path[i+1]] for i in range(len(path) - 1)])
all_paths.append(path)
all_path_lengths.append(path_length)
# 更新信息素
shortest_path_idx = np.argmin(all_path_lengths)
shortest_path = all_paths[shortest_path_idx]
shortest_path_length = all_path_lengths[shortest_path_idx]
for i in range(len(shortest_path) - 1):
pheromone[shortest_path[i]][shortest_path[i+1]] += 1 / shortest_path_length
pheromone *= (1 - decay)
# 输出最优路径
print("最短路径为:", shortest_path)
print("路径长度为:", shortest_path_length)
```
请注意,上述代码是一个非常简化的版本,仅用于演示蚁群算法在路径优化问题中的基本思路。在实际应用中,算法需要根据具体问题进行调整和优化,以达到更好的效果。例如,可以加入对特定约束条件的考虑,如时间窗口限制、车辆容量限制等。
阅读全文
相关推荐
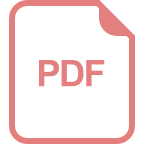
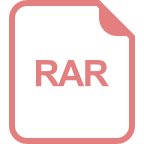
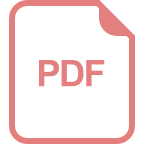
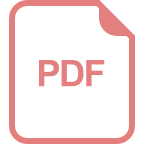
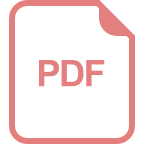
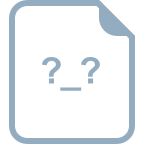
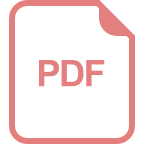
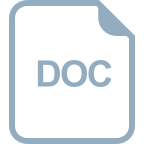
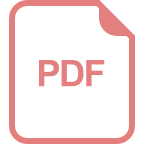
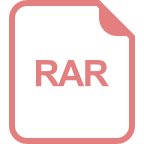
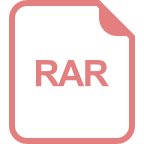
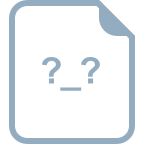
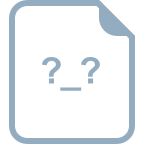
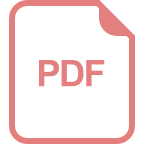
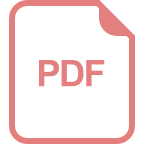