write a epoll udp sample.
时间: 2024-01-15 16:05:02 浏览: 29
Sure, here's a sample code for a UDP server using epoll in C:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <fcntl.h>
#include <sys/epoll.h>
#define MAX_EVENTS 10
#define BUF_SIZE 1024
#define PORT 12345
int main(int argc, char* argv[])
{
int epoll_fd, udp_fd, nfds;
struct epoll_event event, events[MAX_EVENTS];
struct sockaddr_in server_addr, client_addr;
socklen_t addrlen = sizeof(client_addr);
char buf[BUF_SIZE];
// create UDP socket
udp_fd = socket(AF_INET, SOCK_DGRAM, 0);
if (udp_fd == -1) {
perror("socket");
exit(EXIT_FAILURE);
}
// bind the socket to a port
memset(&server_addr, 0, sizeof(server_addr));
server_addr.sin_family = AF_INET;
server_addr.sin_addr.s_addr = htonl(INADDR_ANY);
server_addr.sin_port = htons(PORT);
if (bind(udp_fd, (struct sockaddr*)&server_addr, sizeof(server_addr)) == -1) {
perror("bind");
exit(EXIT_FAILURE);
}
// set socket to non-blocking mode
int flags = fcntl(udp_fd, F_GETFL, 0);
if (flags == -1) {
perror("fcntl");
exit(EXIT_FAILURE);
}
flags |= O_NONBLOCK;
if (fcntl(udp_fd, F_SETFL, flags) == -1) {
perror("fcntl");
exit(EXIT_FAILURE);
}
// create an epoll instance
epoll_fd = epoll_create1(0);
if (epoll_fd == -1) {
perror("epoll_create1");
exit(EXIT_FAILURE);
}
// add the UDP socket to the epoll instance
event.events = EPOLLIN | EPOLLET;
event.data.fd = udp_fd;
if (epoll_ctl(epoll_fd, EPOLL_CTL_ADD, udp_fd, &event) == -1) {
perror("epoll_ctl");
exit(EXIT_FAILURE);
}
while (1) {
nfds = epoll_wait(epoll_fd, events, MAX_EVENTS, -1);
if (nfds == -1) {
perror("epoll_wait");
exit(EXIT_FAILURE);
}
for (int i = 0; i < nfds; i++) {
if (events[i].data.fd == udp_fd) {
// receive data from the UDP socket
int nbytes = recvfrom(udp_fd, buf, BUF_SIZE, 0, (struct sockaddr*)&client_addr, &addrlen);
if (nbytes == -1) {
if (errno == EAGAIN || errno == EWOULDBLOCK) {
// no more data to receive
continue;
} else {
perror("recvfrom");
exit(EXIT_FAILURE);
}
} else {
// send data back to the client
if (sendto(udp_fd, buf, nbytes, 0, (struct sockaddr*)&client_addr, addrlen) == -1) {
perror("sendto");
exit(EXIT_FAILURE);
}
}
}
}
}
return 0;
}
```
This code creates a UDP socket and binds it to a port. It then sets the socket to non-blocking mode and creates an epoll instance. The UDP socket is added to the epoll instance and the code enters a loop that waits for events using `epoll_wait()`. When data is received on the UDP socket, the code sends it back to the client.
相关推荐
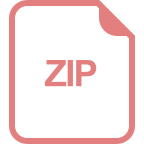
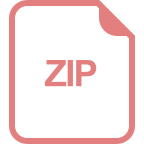














