设计一程序,可以持续输入学生(最多十位)的分数,并可以计算个人的平均分数,各科的平均分数,以及全体的总平均。
时间: 2024-03-01 18:49:36 浏览: 85
以下是 Java 代码实现持续输入学生分数并计算平均分数的过程:
```java
import java.util.Scanner;
public class ScoreCalculator {
public static void main(String[] args) {
final int MAX_STUDENTS = 10; // 最多十位学生
final int SUBJECTS = 3; // 三门科目
int[][] scores = new int[MAX_STUDENTS][SUBJECTS]; // 定义二维数组存储学生分数
int numOfStudents = 0; // 记录输入的学生数
Scanner scanner = new Scanner(System.in);
// 持续输入学生分数
while (numOfStudents < MAX_STUDENTS) {
System.out.print("请输入第" + (numOfStudents + 1) + "位学生的分数(格式:分数1 分数2 分数3):");
String input = scanner.nextLine();
String[] scoresStr = input.split(" ");
if (scoresStr.length != SUBJECTS) {
System.out.println("输入格式错误,请重新输入。");
continue;
}
// 将分数转换为整数并存储到数组中
for (int i = 0; i < SUBJECTS; i++) {
try {
scores[numOfStudents][i] = Integer.parseInt(scoresStr[i]);
} catch (NumberFormatException e) {
System.out.println("输入格式错误,请重新输入。");
continue;
}
}
numOfStudents++;
System.out.println("学生分数已保存。");
System.out.println();
}
// 计算各个学生的平均分数并输出
for (int i = 0; i < numOfStudents; i++) {
int sum = 0;
for (int j = 0; j < SUBJECTS; j++) {
sum += scores[i][j];
}
double average = (double) sum / SUBJECTS;
System.out.println("第" + (i + 1) + "位学生的平均分数为:" + average);
}
// 计算各门科目的平均分数并输出
for (int i = 0; i < SUBJECTS; i++) {
int sum = 0;
for (int j = 0; j < numOfStudents; j++) {
sum += scores[j][i];
}
double average = (double) sum / numOfStudents;
System.out.println("第" + (i + 1) + "门科目的平均分数为:" + average);
}
// 计算全体学生的总平均分数并输出
int sum = 0;
for (int i = 0; i < numOfStudents; i++) {
for (int j = 0; j < SUBJECTS; j++) {
sum += scores[i][j];
}
}
double average = (double) sum / (numOfStudents * SUBJECTS);
System.out.println("全体学生的总平均分数为:" + average);
}
}
```
运行程序后,按照提示输入每位学生的分数,程序将会依次输出每位学生的平均分数、各门科目的平均分数以及全体学生的总平均分数。
阅读全文
相关推荐
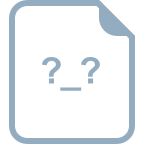
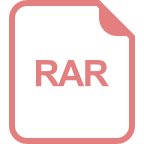
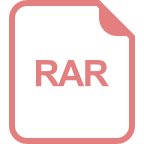
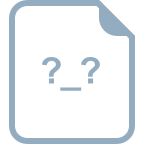
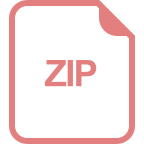
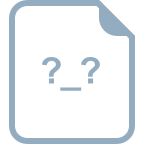
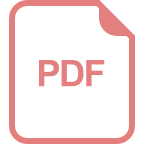
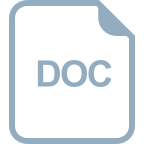
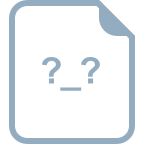
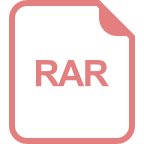
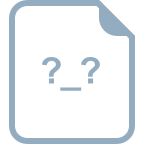
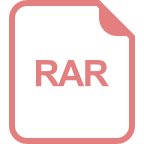
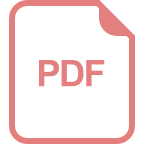
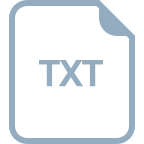
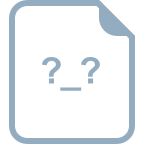
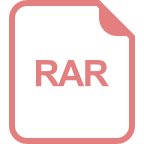
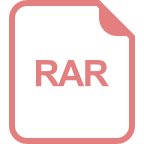
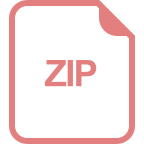