Python编写一个日期类,拥有年月日的数据,定义Myate__init__函数,实现对象的初始化,在数据合理时抛出异常
时间: 2024-05-16 17:18:36 浏览: 20
以下是一个简单的日期类的实现,包含了年月日的数据和一些常用的方法,同时在初始化时会检查日期是否合法,如果不合法则抛出异常。
```python
class Date:
def __init__(self, year, month, day):
self.year = year
self.month = month
self.day = day
if not self._is_valid():
raise ValueError("Invalid date")
def _is_valid(self):
if self.month < 1 or self.month > 12:
return False
if self.day < 1 or self.day > 31:
return False
if self.month == 2:
if self.year % 4 == 0 and (self.year % 100 != 0 or self.year % 400 == 0):
if self.day > 29:
return False
else:
if self.day > 28:
return False
elif self.month in [4, 6, 9, 11]:
if self.day > 30:
return False
return True
def __str__(self):
return f"{self.year}-{self.month:02d}-{self.day:02d}"
def add_days(self, days):
days_in_month = [0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if self.month == 2 and self.year % 4 == 0 and (self.year % 100 != 0 or self.year % 400 == 0):
days_in_month[2] = 29
while days > 0:
days_in_month[self.month] -= self.day
self.day = 0
days_in_month[self.month] += 1
if days > days_in_month[self.month]:
days -= days_in_month[self.month]
self.month += 1
if self.month > 12:
self.month = 1
self.year += 1
if self.month == 2 and self.year % 4 == 0 and (self.year % 100 != 0 or self.year % 400 == 0):
days_in_month[2] = 29
else:
days_in_month[2] = 28
else:
self.day += days
days = 0
```
这个日期类实现了以下功能:
- 初始化时检查日期是否合法,如果不合法则抛出异常
- 提供了一个 `__str__` 方法,用于将日期对象转换成字符串
- 提供了一个 `add_days` 方法,用于增加指定的天数到日期对象中
下面是一个使用示例:
```python
d = Date(2021, 6, 30)
print(d) # 输出: 2021-06-30
d.add_days(5)
print(d) # 输出: 2021-07-05
```
相关推荐
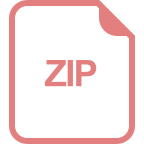
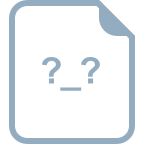
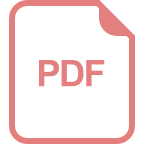














