电文加密的算法 c语言代码
时间: 2024-10-05 21:04:48 浏览: 55
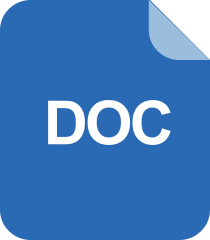
GPS接收机导航电文解调算法实现-正文.doc

在C语言中,我们可以使用简单的凯撒密码(Caesar Cipher)作为电文加密的一个基本示例。凯撒密码是一种替换式加密方法,通过将明文字符向字母表右移固定数量的位置来加密。以下是一个简单的凯撒密码实现:
```c
#include <stdio.h>
#include <string.h>
char encrypt(char c, int shift) {
// 'A'到'Z'的ASCII值范围是65到90
if ('A' <= c && c <= 'Z') {
return ((c - 'A' + shift) % 26) + 'A';
} else if ('a' <= c && c <= 'z') {
return ((c - 'a' + shift) % 26) + 'a';
}
// 对于非字母字符不做处理
return c;
}
void caesar_cipher_encrypt(char *plaintext, char *ciphertext, int shift) {
for (int i = 0; plaintext[i] != '\0'; ++i) {
ciphertext[i] = encrypt(plaintext[i], shift);
}
ciphertext[i] = '\0'; // 结束字符串
}
int main() {
char plaintext[] = "Hello, World!";
char ciphertext[100];
int shift = 3; // 加密偏移量
caesar_cipher_encrypt(plaintext, ciphertext, shift);
printf("原文: %s\n", plaintext);
printf("密文: %s\n", ciphertext);
return 0;
}
```
在这个例子中,`encrypt`函数负责加密单个字符,而`caesar_cipher_encrypt`函数则遍历整个输入字符串并应用加密。你可以根据需要调整`shift`变量来改变加密强度。
阅读全文
相关推荐
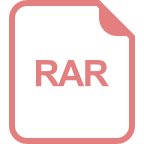
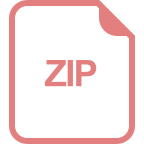


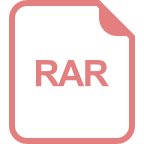








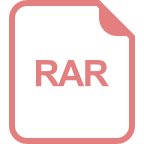