如何手动实现一个单词首字母大写的函数?
时间: 2024-12-14 14:27:44 浏览: 19
手动实现一个单词首字母大写的函数通常涉及到字符串操作,下面是一个简单的Python示例:
```python
def capitalize_first_letter(sentence):
# 将句子拆分成单词列表
words = sentence.split()
# 对每个单词的首字母转为大写,并将其余字符保持原样
capitalized_words = [word[0].upper() + word[1:] for word in words]
# 重新组合成完整的句子
capitalized_sentence = ' '.join(capitalized_words)
return capitalized_sentence
# 使用示例
sentence = "hello world"
capitalized_sentence = capitalize_first_letter(sentence)
print(capitalized_sentence) # 输出:Hello World
```
这个函数通过遍历输入的句子,对每个单词的第一个字符应用`upper()`方法使其变为大写,然后连接所有单词得到结果。
相关问题
单词首字母大写c语言
在C语言中,虽然没有内置的功能强制某个单词的首字母自动大写,但你可以通过手动操作字符串来实现这个需求。通常的做法是在拼接字符串时,如果单词不是第一个单词,就检查前一个字符是否为空格或其他分隔符,如果是,则将当前单词的首字母转换为大写。
例如,可以编写一个简单的函数,接收一个指针作为输入,遍历并处理字符串:
```c
#include <stdio.h>
#include <ctype.h> // 包含对字母大小写的处理函数
void capitalize_first_letter(char *str) {
if (*str != '\0') { // 如果字符串非空
if (isspace(str[1])) { // 检查是不是单词首字母
str[0] = toupper(str[0]); // 转换为大写
}
capitalize_first_letter(&str[1]); // 继续处理下一个单词
}
}
int main() {
char sentence[] = "hello world, this is a test.";
capitalize_first_letter(sentence);
printf("%s", sentence); // 输出:Hello World, This Is A Test.
return 0;
}
```
给定一个字符串,编写一个程序来进行大小写转换,并将字符串中的每个单词的首字母大写python
在Python中,你可以使用内置的`title()`函数来完成字符串的标题化,也就是将每个单词的首字母大写。如果你想自己编写一个简单的函数,可以遍历每个字符并检查是否为单词的开头,如果是,则将其转换为大写。以下是这两种方法的示例:
1. 使用内置 `title()` 函数:
```python
def title_case(s):
return s.title()
input_string = "hello world"
converted_string = title_case(input_string)
print(converted_string) # 输出: Hello World
```
2. 手动实现转换:
```python
def manual_title_case(s):
words = s.split(' ')
capitalized_words = [word[0].upper() + word[1:] for word in words]
return ' '.join(capitalized_words)
input_string = "hello world"
converted_string_manual = manual_title_case(input_string)
print(converted_string_manual) # 输出: Hello World
```
阅读全文
相关推荐






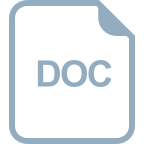









