c++怎样遍历map
时间: 2024-02-25 09:50:08 浏览: 106
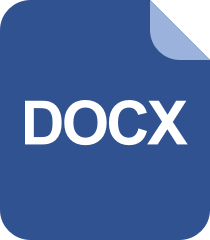
Map遍历方法
在C++中,可以使用迭代器来遍历map。以下是一种常见的遍历map的方法:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "apple";
myMap[2] = "banana";
myMap[3] = "orange";
// 使用迭代器遍历map
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
return 0;
}
```
在上面的示例中,我们首先创建了一个`std::map`对象`myMap`,并向其中插入了几个键值对。然后,我们使用`begin()`和`end()`函数获取map的起始和结束迭代器,并使用一个循环来遍历整个map。在循环中,我们通过迭代器访问每个键值对,并打印出键和值。
注意,上述示例中使用了`auto`关键字来自动推导迭代器的类型,这是C++11引入的特性。如果你使用的是较早的C++版本,可以将`auto`替换为适当的迭代器类型,例如`std::map<int, std::string>::iterator`。
阅读全文
相关推荐
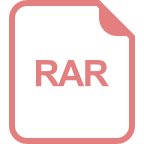















