c语言编写一个控制台应用程序,在主线程中创建两个子线程,在子线程中按一定时间间隔输出提示信息。
时间: 2024-10-20 17:08:09 浏览: 31
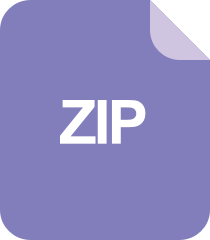
基于C语言实现的(控制台)用户级线程库【100010648】
在 C 语言中,你可以使用 POSIX 线程库(pthread.h)来创建和管理线程。下面是一个简单的示例,展示了如何在主线程中创建两个子线程,并让它们按照指定的时间间隔打印提示信息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#define DELAY_SECONDS 1
void *thread_func(void *arg) {
int i;
for (i = 0; ; i++) {
printf("Thread %d: Hello from child thread %d\n", pthread_self(), i);
sleep(DELAY_SECONDS); // 暂停一段时间
if (i >= 5) { // 如果超过5次循环就退出
break;
}
}
return NULL;
}
int main() {
pthread_t thread1, thread2; // 创建两个线程变量
int rc;
// 创建第一个线程
rc = pthread_create(&thread1, NULL, thread_func, NULL);
if (rc) {
fprintf(stderr, "Error creating thread 1: %d\n", rc);
return -1;
}
// 创建第二个线程
rc = pthread_create(&thread2, NULL, thread_func, NULL);
if (rc) {
fprintf(stderr, "Error creating thread 2: %d\n", rc);
pthread_cancel(thread1); // 如果创建失败尝试取消第一个线程
pthread_join(thread1, NULL);
return -1;
}
// 主线程等待子线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("Main thread: Both child threads finished.\n");
return 0;
}
```
在这个例子中,`thread_func` 是每个子线程的任务,它会无限循环地输出消息并暂停指定秒数。在 `main` 函数里,我们使用 `pthread_create` 来创建线程,然后通过 `pthread_join` 来等待子线程完成。
注意:这个程序假设了 `sleep` 和 `printf` 的同步机制,实际应用可能需要处理线程间的同步问题,比如使用互斥锁(mutex)来避免数据竞争。
阅读全文
相关推荐
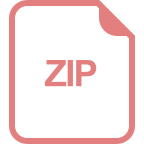


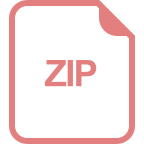
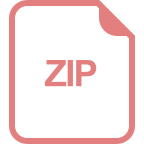
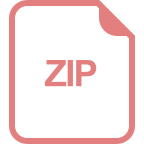




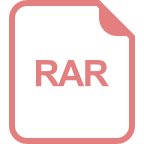
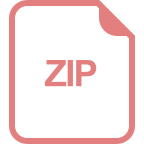
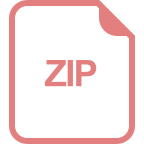
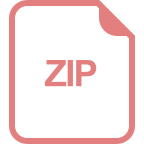
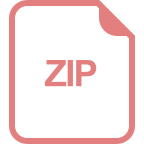