二叉树基本操作演示程序 设计一个与二叉树基本操作相关的演示程序。 要求: (1)创建二叉树。按照用户需要的二叉树,构建二叉树。 (2)分别以先序、中序、后序三种遍历访问二叉树。 (3)输出二叉树的叶子结点及叶子结点的个数。 (4)输出二叉树的高度。
时间: 2024-05-12 10:12:16 浏览: 67
以下是二叉树基本操作的演示程序,程序中包括二叉树的创建、三种遍历方式、叶子节点个数和高度的计算:
```
#include <iostream>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
};
void buildTree(TreeNode* &root) {
int val;
cout << "请输入结点的值(输入-1表示该结点为空):";
cin >> val;
if (val == -1) {
root = nullptr;
return;
}
root = new TreeNode(val);
buildTree(root->left);
buildTree(root->right);
}
void preOrder(TreeNode* root) {
if (root == nullptr) return;
cout << root->val << " ";
preOrder(root->left);
preOrder(root->right);
}
void inOrder(TreeNode* root) {
if (root == nullptr) return;
inOrder(root->left);
cout << root->val << " ";
inOrder(root->right);
}
void postOrder(TreeNode* root) {
if (root == nullptr) return;
postOrder(root->left);
postOrder(root->right);
cout << root->val << " ";
}
int leafCount(TreeNode* root) {
if (root == nullptr) return 0;
if (root->left == nullptr && root->right == nullptr) return 1;
return leafCount(root->left) + leafCount(root->right);
}
int getHeight(TreeNode* root) {
if (root == nullptr) return 0;
return max(getHeight(root->left), getHeight(root->right)) + 1;
}
int main() {
TreeNode* root = nullptr;
buildTree(root);
cout << "先序遍历结果为:";
preOrder(root);
cout << endl;
cout << "中序遍历结果为:";
inOrder(root);
cout << endl;
cout << "后序遍历结果为:";
postOrder(root);
cout << endl;
int count = leafCount(root);
cout << "叶子节点个数为:" << count << endl;
int height = getHeight(root);
cout << "二叉树的高度为:" << height << endl;
return 0;
}
```
阅读全文
相关推荐
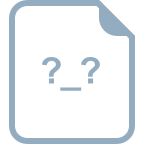
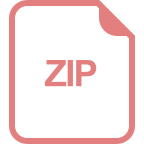
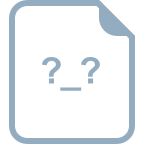
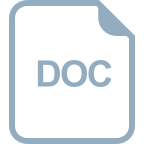
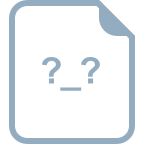
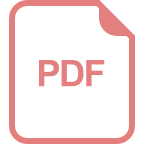

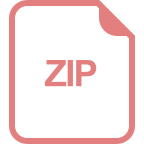
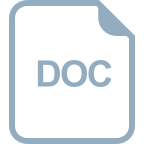
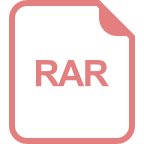
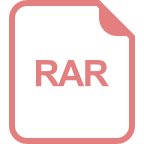
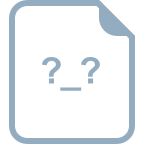
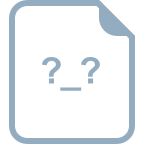
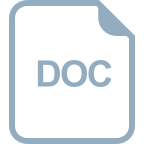
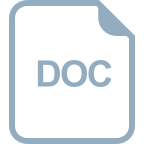
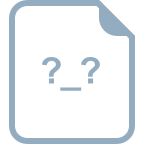