Digest认证的接口工具类怎么写
时间: 2024-09-14 09:16:44 浏览: 35
Digest认证是一种HTTP基本认证的扩展形式,通常用于保护RESTful API。在编写Digest认证的接口工具类时,你需要考虑以下几个步骤:
1. **导入依赖**:
首先需要导入相关的库,如`java.net.HttpURLConnection`、`java.security.MessageDigest`等。
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.security.MessageDigest;
```
2. **创建请求头**:
创建包含Authorization字段的请求头,其中包含了计算出的摘要信息。这通常涉及到用户的用户名和密码以及特定的算法(如MD5或SHA-1)。
```java
public static String createDigestAuthHeader(String username, String password, String algorithm) {
try {
MessageDigest digest = MessageDigest.getInstance(algorithm);
byte[] credentials = (username + ":" + password).getBytes("UTF-8");
byte[] digestBytes = digest.digest(credentials);
StringBuilder hex = new StringBuilder();
for (byte b : digestBytes) {
hex.append(String.format("%02x", b & 0xff));
}
return "Basic " + Base64.getEncoder().encodeToString(hex.toString().getBytes());
} catch (Exception e) {
throw new RuntimeException("Failed to generate Digest authentication header", e);
}
}
```
3. **发送请求并处理响应**:
使用`HttpURLConnection`发送GET或POST请求,并设置正确的请求头。
```java
public HttpURLConnection makeAuthenticatedRequest(URL url, String username, String password, String algorithm) throws Exception {
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET"); // 替换成实际需求的请求方法
conn.setRequestProperty("Authorization", createDigestAuthHeader(username, password, algorithm));
conn.setDoOutput(true); // 如果有需要,可以设置输出流
int responseCode = conn.getResponseCode();
if (responseCode != HttpURLConnection.HTTP_OK) {
throw new RuntimeException("Authentication failed: " + responseCode);
}
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
// 读取并处理服务器返回的内容
String line;
StringBuilder content = new StringBuilder();
while ((line = reader.readLine()) != null) {
content.append(line);
}
reader.close();
return conn;
}
```
阅读全文
相关推荐
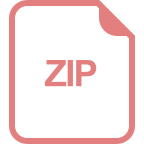
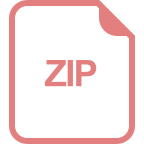
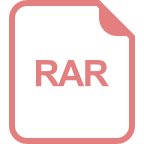
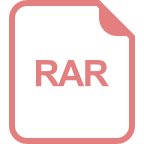
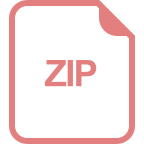
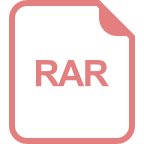
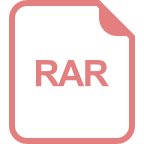
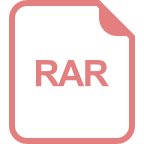
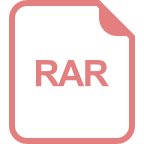
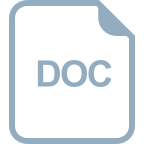
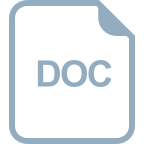
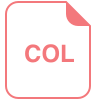
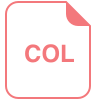
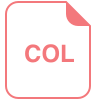
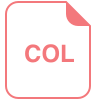
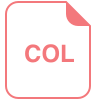
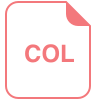
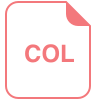
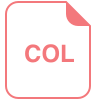