c语言写一个贪吃蛇游戏代码
时间: 2023-06-18 13:07:43 浏览: 77
以下是一个基本的C语言贪吃蛇游戏代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#include <windows.h>
#define WIDTH 30 // 游戏界面宽度
#define HEIGHT 20 // 游戏界面高度
int snake[WIDTH * HEIGHT][2]; // 蛇身
int food[2]; // 食物
int score = 0; // 得分
int direction = 2; // 蛇头方向,初始向右
int speed = 200; // 蛇移动速度,单位毫秒
void gotoxy(int x, int y) {
COORD c;
c.X = x;
c.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), c);
}
void draw() {
system("cls");
for (int i = 0; i <= WIDTH + 1; i++) {
gotoxy(i, 0);
printf("#");
gotoxy(i, HEIGHT + 1);
printf("#");
}
for (int i = 0; i <= HEIGHT + 1; i++) {
gotoxy(0, i);
printf("#");
gotoxy(WIDTH + 1, i);
printf("#");
}
for (int i = 1; i <= score; i++) {
gotoxy(snake[i][0], snake[i][1]);
printf("*");
}
gotoxy(food[0], food[1]);
printf("@");
gotoxy(WIDTH + 3, 2);
printf("Score: %d", score);
}
void init() {
srand(time(NULL));
snake[1][0] = 5;
snake[1][1] = 5;
snake[2][0] = 4;
snake[2][1] = 5;
food[0] = rand() % (WIDTH - 2) + 2;
food[1] = rand() % (HEIGHT - 2) + 2;
draw();
}
void move() {
for (int i = score; i >= 2; i--) {
snake[i][0] = snake[i - 1][0];
snake[i][1] = snake[i - 1][1];
}
switch (direction) {
case 1: // 上
snake[1][1]--;
break;
case 2: // 右
snake[1][0]++;
break;
case 3: // 下
snake[1][1]++;
break;
case 4: // 左
snake[1][0]--;
break;
}
if (snake[1][0] == food[0] && snake[1][1] == food[1]) {
score++;
food[0] = rand() % (WIDTH - 2) + 2;
food[1] = rand() % (HEIGHT - 2) + 2;
}
}
int main() {
init();
while (1) {
if (_kbhit()) {
int ch = _getch();
if (ch == 224) {
ch = _getch();
if ((ch == 72 && direction != 3) || (ch == 80 && direction != 1)
|| (ch == 77 && direction != 4) || (ch == 75 && direction != 2)) {
direction = ch == 72 ? 1 : (ch == 80 ? 3 : (ch == 77 ? 2 : 4));
}
} else if (ch == ' ') {
while (_getch() != ' ');
} else {
break;
}
}
move();
draw();
Sleep(speed);
}
return 0;
}
```
这里使用了Windows API函数`gotoxy`实现控制光标位置输出字符,同时使用了`_kbhit`和`_getch`函数实现键盘输入检测和读取。游戏的核心逻辑在`move`函数中实现,包括蛇的移动、食物的生成和得分的更新。
相关推荐
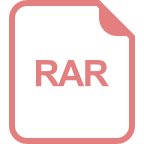







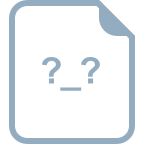
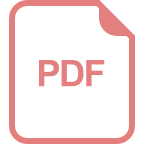
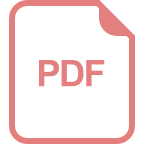
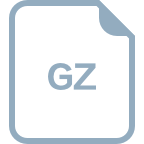