Java 递归反序列化树形JSON数据,生成平级数据
时间: 2023-10-10 14:10:55 浏览: 97
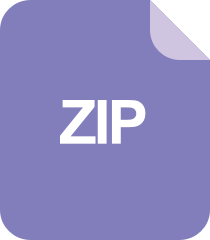
json反序列化
假设我们有以下的树形 JSON 数据:
```json
{
"id": 1,
"name": "A",
"children": [
{
"id": 2,
"name": "B",
"children": [
{
"id": 3,
"name": "C",
"children": []
},
{
"id": 4,
"name": "D",
"children": []
}
]
},
{
"id": 5,
"name": "E",
"children": [
{
"id": 6,
"name": "F",
"children": []
}
]
}
]
}
```
现在我们想要将它转换为平级数据,即:
```
1-A
2-B
3-C
4-D
5-E
6-F
```
我们可以使用递归的方式来实现这个功能,具体的步骤如下:
1. 定义一个 `TreeNode` 类来表示树形节点。
```java
public class TreeNode {
private int id;
private String name;
private List<TreeNode> children;
public TreeNode(int id, String name, List<TreeNode> children) {
this.id = id;
this.name = name;
this.children = children;
}
// getters and setters
}
```
2. 编写递归函数 `flatten`,用于将树形结构转换为平级结构。
```java
public static List<String> flatten(TreeNode node) {
List<String> result = new ArrayList<>();
result.add(node.getId() + "-" + node.getName());
for (TreeNode child : node.getChildren()) {
result.addAll(flatten(child));
}
return result;
}
```
3. 在主函数中调用 `flatten` 函数,并将结果输出。
```java
public static void main(String[] args) {
String json = "{\"id\":1,\"name\":\"A\",\"children\":[{\"id\":2,\"name\":\"B\",\"children\":[{\"id\":3,\"name\":\"C\",\"children\":[]},{\"id\":4,\"name\":\"D\",\"children\":[]}]},{\"id\":5,\"name\":\"E\",\"children\":[{\"id\":6,\"name\":\"F\",\"children\":[]}]}]}";
Gson gson = new Gson();
TreeNode root = gson.fromJson(json, TreeNode.class);
List<String> result = flatten(root);
for (String s : result) {
System.out.println(s);
}
}
```
运行程序后,输出以下结果:
```
1-A
2-B
3-C
4-D
5-E
6-F
```
这样我们就成功地将树形 JSON 数据转换为平级数据了。
阅读全文
相关推荐
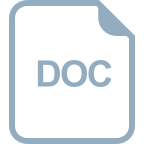
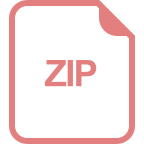
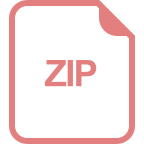
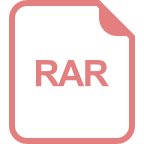
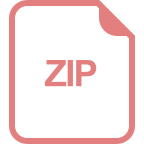
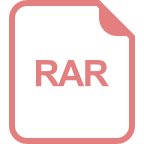
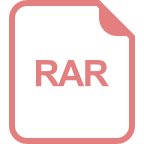
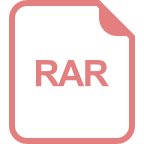
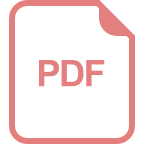
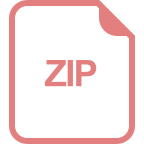
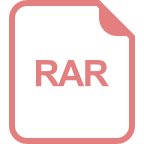
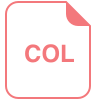
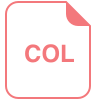
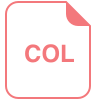
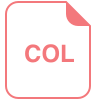
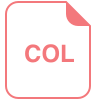