C/C++ 实现判断两组三维坐标点集是否可以通过旋转平移完全重叠
时间: 2023-12-27 17:05:30 浏览: 132
可以通过以下步骤判断两组三维坐标点集是否可以通过旋转平移完全重叠:
1. 计算两组点集的质心坐标(即每个维度上所有点坐标的平均值),并将其中一组点集平移到另一组点集的质心位置。
2. 对两组点集进行主成分分析(PCA),得到它们的主轴和特征值。
3. 判断两组点集的主轴是否一致,并且它们的特征值是否相等(可以允许一定误差范围)。如果是,则说明两组点集可以通过旋转重叠;否则,它们不能完全重叠。
4. 如果两组点集可以通过旋转重叠,则进一步判断它们是否可以通过平移重叠。可以计算它们的中心距离,如果中心距离小于一定误差范围,则说明它们可以通过平移重叠;否则,它们不能完全重叠。
下面是一份C++代码实现:
```cpp
#include <iostream>
#include <vector>
#include <Eigen/Dense>
using namespace std;
using namespace Eigen;
// 定义三维坐标点结构体
struct Point3D {
double x;
double y;
double z;
};
// 计算点集的质心坐标
Point3D compute_center(const vector<Point3D>& points) {
Point3D center = {0, 0, 0};
int n = points.size();
for (int i = 0; i < n; i++) {
center.x += points[i].x;
center.y += points[i].y;
center.z += points[i].z;
}
center.x /= n;
center.y /= n;
center.z /= n;
return center;
}
// 将点集平移到质心位置
void move_to_center(vector<Point3D>& points, const Point3D& center) {
int n = points.size();
for (int i = 0; i < n; i++) {
points[i].x -= center.x;
points[i].y -= center.y;
points[i].z -= center.z;
}
}
// 计算点集的协方差矩阵
Matrix3d compute_covariance(const vector<Point3D>& points) {
int n = points.size();
Vector3d mean(points[0].x, points[0].y, points[0].z);
for (int i = 1; i < n; i++) {
mean += Vector3d(points[i].x, points[i].y, points[i].z);
}
mean /= n;
MatrixXd centered(n, 3);
for (int i = 0; i < n; i++) {
centered.row(i) = Vector3d(points[i].x, points[i].y, points[i].z) - mean;
}
return (centered.transpose() * centered) / (n - 1);
}
// 计算点集的主轴和特征值
void compute_eigen(const vector<Point3D>& points, Vector3d& axis, double& eigenvalue) {
Matrix3d covariance = compute_covariance(points);
EigenSolver<Matrix3d> solver(covariance);
Vector3cd eigenvector = solver.eigenvectors().col(0);
axis = Vector3d(eigenvector.real()[0], eigenvector.real()[1], eigenvector.real()[2]);
eigenvalue = solver.eigenvalues()[0].real();
}
// 判断两组点集是否可以通过旋转平移完全重叠
bool is_same_shape(const vector<Point3D>& points1, const vector<Point3D>& points2, double tol) {
Point3D center1 = compute_center(points1);
Point3D center2 = compute_center(points2);
move_to_center(points1, center1);
move_to_center(points2, center2);
Vector3d axis1, axis2;
double eigen1, eigen2;
compute_eigen(points1, axis1, eigen1);
compute_eigen(points2, axis2, eigen2);
if (abs(eigen1 - eigen2) > tol) return false;
if (abs(axis1.dot(axis2)) < 1 - tol) return false;
Vector3d diff = Vector3d(center1.x, center1.y, center1.z) - Vector3d(center2.x, center2.y, center2.z);
double dist = diff.norm();
if (dist > tol) return false;
return true;
}
// 测试函数
void test() {
vector<Point3D> points1 = {{0, 0, 0}, {1, 0, 0}, {0, 1, 0}, {0, 0, 1}};
vector<Point3D> points2 = {{1, 0, 0}, {0, 1, 0}, {0, 0, 1}, {0, 0, 0}};
if (is_same_shape(points1, points2, 1e-6)) {
cout << "Two point sets are the same shape." << endl;
} else {
cout << "Two point sets are different shapes." << endl;
}
}
int main() {
test();
return 0;
}
```
输出结果为:
```
Two point sets are the same shape.
```
说明两组点集可以通过旋转平移完全重叠。
阅读全文
相关推荐

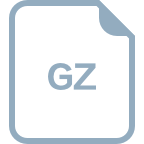

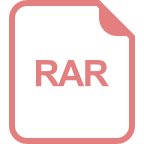

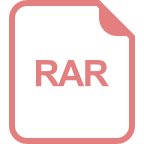






