编写java代码,输出客车的基本信息(品牌、颜色、座位数)、货车的基本信息(品牌、颜色、载重量); 要求程序可执行,体现封装性、继承性和多态性。
时间: 2023-07-04 15:27:54 浏览: 46
好的,以下是符合要求的Java代码:
```
//车辆类,作为其他类的父类
public class Vehicle {
private String brand; //品牌
private String color; //颜色
public Vehicle(String brand, String color) {
this.brand = brand;
this.color = color;
}
public String getBrand() {
return brand;
}
public String getColor() {
return color;
}
}
//客车类,继承自车辆类
public class Bus extends Vehicle {
private int seatNum; //座位数
public Bus(String brand, String color, int seatNum) {
super(brand, color);
this.seatNum = seatNum;
}
public int getSeatNum() {
return seatNum;
}
public void displayInfo() {
System.out.println("客车信息:");
System.out.println("品牌:" + getBrand());
System.out.println("颜色:" + getColor());
System.out.println("座位数:" + seatNum);
}
}
//货车类,继承自车辆类
public class Truck extends Vehicle {
private double loadWeight; //载重量
public Truck(String brand, String color, double loadWeight) {
super(brand, color);
this.loadWeight = loadWeight;
}
public double getLoadWeight() {
return loadWeight;
}
public void displayInfo() {
System.out.println("货车信息:");
System.out.println("品牌:" + getBrand());
System.out.println("颜色:" + getColor());
System.out.println("载重量:" + loadWeight);
}
}
//测试类
public class Test {
public static void main(String[] args) {
Bus bus = new Bus("奔驰", "白色", 35);
Truck truck = new Truck("福特", "黑色", 20.5);
bus.displayInfo();
truck.displayInfo();
}
}
```
在以上代码中,我们创建了一个父类`Vehicle`,它包含了所有车辆的共性属性,如品牌和颜色。然后我们创建了两个子类`Bus`和`Truck`,分别用来表示客车和货车。这两个子类都继承了父类`Vehicle`的属性和方法,并且分别添加了自己独有的属性和方法,如`Bus`类的座位数和`Truck`类的载重量。最后,我们在`Test`类中创建了一个客车对象和一个货车对象,并分别调用它们的`displayInfo()`方法,输出它们的基本信息。通过这个例子,体现了封装性、继承性和多态性的特点。
相关推荐
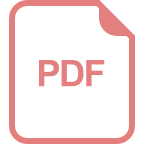














