用C语言写一个 从键盘上输入一个四则运算的式子并输出计算结果
时间: 2023-08-31 11:18:53 浏览: 123
### 回答1:
首先,可以使用 scanf 函数从键盘输入一个字符串,然后使用栈来处理这个字符串并计算表达式的结果。
代码示例如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
// 定义栈结构体
typedef struct {
double data[MAX_STACK_SIZE];
int top;
} Stack;
// 初始化栈
void init_stack(Stack* stack) {
stack->top = -1;
}
// 判断栈是否为空
int is_empty(Stack* stack) {
return stack->top == -1;
}
// 判断栈是否已满
int is_full(Stack* stack) {
return stack->top == MAX_STACK_SIZE - 1;
}
// 入栈
void push(Stack* stack, double value) {
if (is_full(stack)) {
printf("Error: stack is full.\n");
exit(1);
}
stack->data[++stack->top] = value;
}
// 出栈
double pop(Stack* stack) {
if (is_empty(stack)) {
printf("Error: stack is empty.\n");
exit(1);
}
return stack->data[stack->top--];
}
// 获取栈顶元素
double peek(Stack* stack) {
if (is_empty(stack)) {
printf("Error: stack is empty.\n");
exit(1);
}
return stack->data[stack->top];
}
int main(int argc, char* argv[]) {
char expression[MAX_STACK_SIZE]; // 定义一个字符数组来存储表达式
printf("Please enter an arithmetic expression: ");
scanf("%s", expression); // 从键盘输入表达式
Stack operand_stack; // 定义一个操作数栈
init_stack(&operand_stack); // 初始化操作数栈
Stack operator_stack; // 定义一个操作符栈
init_stack(&operator_stack); // 初始化操作符栈
// 遍历表达式中的每个字
### 回答2:
好的,以下是用C语言写的一个从键盘上输入一个四则运算的式子并输出计算结果的简单示例:
```c
#include <stdio.h>
int main() {
float num1, num2, result;
char operator;
printf("请输入一个四则运算的式子(例如2 + 3):");
scanf("%f %c %f", &num1, &operator, &num2);
switch(operator) {
case '+':
result = num1 + num2;
printf("结果为 %.2f\n", result);
break;
case '-':
result = num1 - num2;
printf("结果为 %.2f\n", result);
break;
case '*':
result = num1 * num2;
printf("结果为 %.2f\n", result);
break;
case '/':
if(num2 != 0) {
result = num1 / num2;
printf("结果为 %.2f\n", result);
} else {
printf("错误:除数不能为0\n");
}
break;
default:
printf("错误:无效的运算符\n");
break;
}
return 0;
}
```
这段代码首先提示用户输入一个四则运算的式子,然后使用`scanf`函数按照格式`%f %c %f`读取用户输入的两个数和一个运算符。接着使用`switch`语句判断运算符的类型,并根据相应的情况进行计算,最后输出结果。如果用户输入的是除法,还会进行除数为0的判断。
注意,以上示例代码假设用户输入的是两个浮点数,运算结果也会是浮点数,并且结果会被格式化为保留两位小数的形式进行输出。如果需要输入整数,可以将`%f`改为`%d`,相应地修改数据类型及格式化输出的部分即可。
### 回答3:
使用C语言编写一个程序,实现从键盘上输入一个包含四则运算符的算术表达式,并输出计算结果。以下是一个简单的实现示例:
```c
#include <stdio.h>
int main() {
float num1, num2, result;
char operator;
printf("请输入一个四则运算表达式(例如2+3):");
scanf("%f%c%f", &num1, &operator, &num2);
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 != 0) {
result = num1 / num2;
} else {
printf("除数不能为0。\n");
return 0;
}
break;
default:
printf("无效的运算符。\n");
return 0;
}
printf("计算结果为:%f\n", result);
return 0;
}
```
以上程序首先提示用户输入一个四则运算表达式,然后使用`scanf`函数按照指定格式读取输入的操作数和运算符。接着,使用`switch`语句根据运算符进行相应的计算操作,并将结果存储在`result`变量中。如果输入的运算符是无效的,会输出相应的错误提示信息。最后,程序输出计算结果。
注意,以上示例假设输入的操作数为浮点型,如果需要支持整数型操作数,请将`float`替换为`int`。
相关推荐
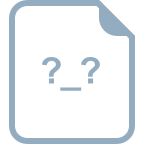
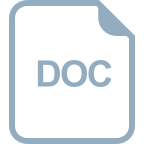
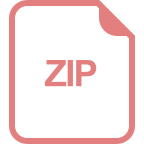

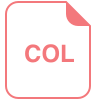
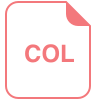
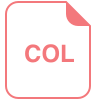
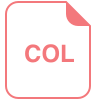









