做一个自定义七段数码管控件,要求小数点显示位数可设置,字体大小,字宽可设置,以及字体颜色,在显示数字时,对应的段亮起,不亮的部分也要通过空白显示出来。给一个尽可能详细的代码并加详细的注释,C#visual studio 2015
时间: 2024-10-25 10:11:23 浏览: 85
创建一个自定义七段数码管控件在C# Visual Studio 2015中需要使用WinForms控件的基础,并结合一些图形处理。以下是一个基本的示例代码,展示了如何实现这个功能:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
// 自定义七段数码管类
public class CustomSevenSegmentDisplay : PictureBox
{
// 数码管的七个段
private const int[] Segments = { 0, 4, 2, 6, 1, 5, 3 };
// 字体属性
public int FontSize { get; set; }
public Color TextColor { get; set; }
// 小数点位置和宽度
public int DecimalPlace { get; set; }
public int DecimalWidth { get; set; }
// 构造函数初始化基本组件
public CustomSevenSegmentDisplay()
{
Font = new Font("Arial", FontSize);
BackColor = Color.Black;
BorderStyle = BorderStyle.None;
}
// 更新显示内容
public void UpdateDisplay(string number)
{
// 清除当前显示
Graphics g = CreateGraphics();
g.Clear(BackColor);
// 设置文本的位置,包括小数点
int x = (Width - Font.Width * number.Length) / 2;
int y = Height / 2 - Font.Height / 2;
// 遍历每个字符和相应的段
for (int i = 0; i < number.Length; i++)
{
char c = number[i];
if (c == '.')
{
// 小数点显示
g.DrawString(".", Font, TextColor, x + i * Font.Width - DecimalWidth / 2, y);
}
else
{
// 大数字符显示
string digitStr = Convert.ToString(c).PadLeft(2, '0');
for (int j = 0; j < 7; j++)
{
if ((digitStr[j] - '0') % 2 == Segments[j])
{
// 对应的段点亮
g.FillRectangle(Brushes.White, x + i * Font.Width + j * Font.Width / 7 - 1, y - 1, Font.Width / 7, Font.Height + 2);
}
}
}
}
// 刷新屏幕
Invalidate();
}
}
// 使用示例
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
// 创建自定义数码管控件实例
CustomSevenSegmentDisplay display = new CustomSevenSegmentDisplay();
display.Location = new Point(10, 10);
display.Size = new Size(100, 50); // 指定尺寸
decimalPlaceTextBox.DataBindings.Add("Text", this, "DecimalPlace");
decimalWidthTextBox.DataBindings.Add("Text", this, "DecimalWidth");
Controls.Add(display);
// 更新控件显示
display.UpdateDisplay("123.45"); // 输入数字进行显示
}
// 监听输入框变化更新显示
private void decimalPlaceTextBox_TextChanged(object sender, EventArgs e)
{
decimalPlaceTextBox.DataBindings["Text"].WriteValue();
}
private void decimalWidthTextBox_TextChanged(object sender, EventArgs e)
{
decimalWidthTextBox.DataBindings["Text"].WriteValue();
}
}
```
这个例子中,我们首先创建了一个名为`CustomSevenSegmentDisplay`的自定义PictureBox类,它包含了数字、小数点和字体的相关设置。`UpdateDisplay`方法负责根据传入的字符串绘制到控件上。
在主窗体`MainForm`中,我们实例化了该控件,并绑定了一些输入框来控制小数点的位置和宽度。当这些输入框的内容改变时,会自动更新数码管的显示。
阅读全文
相关推荐



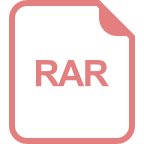
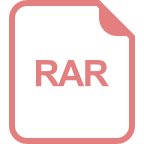



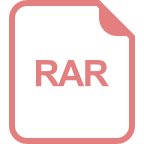
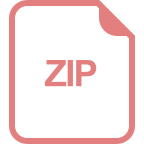


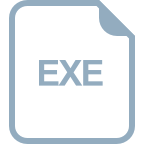
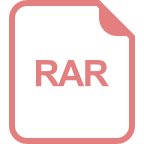
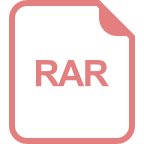
