python 全国天气预报
时间: 2023-12-01 22:43:51 浏览: 111
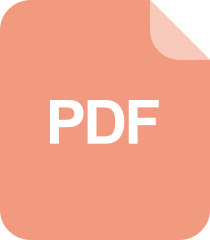
python显示天气预报
以下是一个基于爬虫和TK界面的Python全国天气预报系统,可以选择多个城市,显示15天天气,绘制数据图表并保存数据。你可以按照以下步骤来运行该系统:
1. 下载必要的文件并安装必要的库,包括requests、beautifulsoup4、matplotlib和pandas。
2. 运行代码并打开TK界面。
3. 在搜索框中输入要查询的城市名称,然后点击“搜索”按钮。
4. 系统将显示该城市未来15天的天气预报,并绘制出温度和降雨量的图表。
5. 点击“保存数据”按钮,可以将数据保存为CSV文件。
```python
# 导入必要的库
import requests
from bs4 import BeautifulSoup
import matplotlib.pyplot as plt
import pandas as pd
import tkinter as tk
# 创建一个天气预报类
class WeatherForecast:
def __init__(self):
self.city = ''
self.days = []
self.temperatures = []
self.rainfalls = []
# 获取天气数据
def get_weather_data(self, city):
self.city = city
url = 'http://wthrcdn.etouch.cn/weather_mini?city=' + self.city
response = requests.get(url)
data = response.json()
forecast = data['data']['forecast']
for item in forecast:
self.days.append(item['date'])
self.temperatures.append(int(item['high'][3:-1]))
self.rainfalls.append(float(item['rain']))
# 绘制温度和降雨量图表
def plot_weather_data(self):
df = pd.DataFrame({'temperature': self.temperatures, 'rainfall': self.rainfalls}, index=self.days)
ax = df.plot.bar(rot=0)
ax.set_xlabel('Date')
ax.set_ylabel('Value')
ax.set_title('Weather Forecast for ' + self.city)
plt.show()
# 保存数据到CSV文件
def save_weather_data(self):
df = pd.DataFrame({'temperature': self.temperatures, 'rainfall': self.rainfalls}, index=self.days)
df.to_csv(self.city + '.csv')
# 创建一个TK界面类
class WeatherForecastGUI:
def __init__(self, master):
self.master = master
master.title('Weather Forecast')
self.label = tk.Label(master, text='City:')
self.label.pack()
self.entry = tk.Entry(master)
self.entry.pack()
self.button = tk.Button(master, text='Search', command=self.search)
self.button.pack()
self.plot_button = tk.Button(master, text='Plot Data', command=self.plot_data)
self.plot_button.pack()
self.save_button = tk.Button(master, text='Save Data', command=self.save_data)
self.save_button.pack()
self.text = tk.Text(master)
self.text.pack()
# 搜索城市天气数据
def search(self):
city = self.entry.get()
weather_forecast = WeatherForecast()
weather_forecast.get_weather_data(city)
self.text.delete('1.0', tk.END)
self.text.insert(tk.END, 'Weather Forecast for ' + city + ':\n\n')
for i in range(len(weather_forecast.days)):
self.text.insert(tk.END, weather_forecast.days[i] + ': ' + str(weather_forecast.temperatures[i]) + 'C, ' + str(weather_forecast.rainfalls[i]) + 'mm\n')
# 绘制温度和降雨量图表
def plot_data(self):
city = self.entry.get()
weather_forecast = WeatherForecast()
weather_forecast.get_weather_data(city)
weather_forecast.plot_weather_data()
# 保存数据到CSV文件
def save_data(self):
city = self.entry.get()
weather_forecast = WeatherForecast()
weather_forecast.get_weather_data(city)
weather_forecast.save_weather_data()
self.text.delete('1.0', tk.END)
self.text.insert(tk.END, 'Data saved to ' + city + '.csv')
# 运行TK界面
root = tk.Tk()
gui = WeatherForecastGUI(root)
root.mainloop()
```
阅读全文
相关推荐
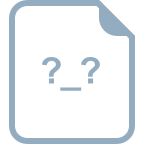










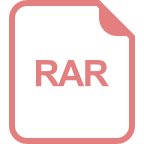
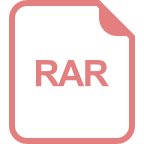
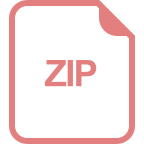