帮我用java语言写一个快速排序
时间: 2023-09-09 19:04:54 浏览: 83
### 回答1:
很高兴能够为您提供帮助。我可以为您提供一个用Java语言编写的快速排序的示例代码:public class QuickSort {
public static void main(String[] args) {
// 声明数组
int[] arr = {10,5,2,3};
// 调用快速排序方法
quickSort(arr, 0, arr.length-1);
// 输出排序后的结果
for(int i:arr){
System.out.print(i+" ");
}
}
// 快速排序
public static void quickSort(int[] arr,int low,int high){
if(low<high){
int index=getIndex(arr,low,high);
quickSort(arr, 0, index-1);
quickSort(arr, index+1, high);
}
}
// 获取分区索引
public static int getIndex(int[] arr,int low,int high){
int temp=arr[low];
while (low<high) {
while(low<high && arr[high]>=temp){//从右向左找
high--;
}
arr[low]=arr[high];
while(low<high && arr[low]<=temp){//从左向右找
low++;
}
arr[high]=arr[low];
}
arr[low]=temp;
return low;
}
}
### 回答2:
快速排序是一种常见的排序算法,它的思想是选取一个基准元素,将数组分成两部分,一部分比基准元素大,一部分比基准元素小,然后对两部分数组递归进行快速排序。
下面是用Java语言实现快速排序的代码:
```java
public class QuickSort {
public static void main(String[] args) {
int[] array = {9, 5, 1, 3, 10, 8, 2, 4, 6, 7};
quickSort(array, 0, array.length - 1);
for (int num : array) {
System.out.print(num + " ");
}
}
public static void quickSort(int[] array, int left, int right) {
if (left < right) {
int pivotIndex = partition(array, left, right); // 获取基准元素的位置
quickSort(array, left, pivotIndex - 1); // 对基准元素的左半部分进行快速排序
quickSort(array, pivotIndex + 1, right); // 对基准元素的右半部分进行快速排序
}
}
public static int partition(int[] array, int left, int right) {
int pivot = array[left]; // 取第一个元素作为基准元素
int i = left;
int j = right;
while (i < j) {
// 从右向左找第一个小于基准元素的位置
while (i < j && array[j] >= pivot) {
j--;
}
if (i < j) {
array[i] = array[j];
i++;
}
// 从左向右找第一个大于基准元素的位置
while (i < j && array[i] <= pivot) {
i++;
}
if (i < j) {
array[j] = array[i];
j--;
}
}
array[i] = pivot; // 将基准元素放入正确的位置
return i; // 返回基准元素的位置
}
}
```
以上代码通过递归调用`quickSort`方法实现快速排序,其中`partition`方法用于获得基准元素的位置,并将数组进行分割。最后,输出排序后的数组。
### 回答3:
快速排序是一种常见的排序算法,它的主要思想是通过将待排序的序列划分为较小和较大的两个子序列,然后递归地对两个子序列进行排序,以达到整个序列有序的目的。以下是使用Java语言实现快速排序的代码示例:
```java
public class QuickSort {
public static void main(String[] args) {
int[] nums = {5, 9, 3, 1, 6, 2, 8, 4, 7};
quickSort(nums, 0, nums.length - 1);
for (int num : nums) {
System.out.print(num + " ");
}
}
public static void quickSort(int[] nums, int low, int high) {
if (low < high) {
int pivot = partition(nums, low, high);
quickSort(nums, low, pivot - 1);
quickSort(nums, pivot + 1, high);
}
}
public static int partition(int[] nums, int low, int high) {
int pivot = nums[low];
while (low < high) {
while (low < high && nums[high] >= pivot) {
high--;
}
nums[low] = nums[high];
while (low < high && nums[low] <= pivot) {
low++;
}
nums[high] = nums[low];
}
nums[low] = pivot;
return low;
}
}
```
在这段代码中,我们定义了一个`quickSort`方法来进行快速排序。首先选择一个枢轴元素(这里选择序列的第一个元素),然后从序列的两端开始向中间遍历,将比枢轴小的元素移到枢轴的左边,将比枢轴大的元素移到枢轴的右边。然后将枢轴放在最终的位置上,分别对左右两个子序列递归执行快速排序。
以上就是使用Java语言实现快速排序的示例代码。
相关推荐
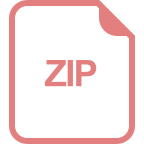
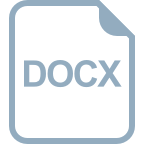
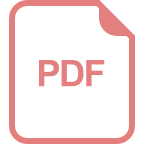











