用C语言设计一个基于图的带权值的邻接矩阵的图的基本操作的演示程序
时间: 2024-02-25 20:53:09 浏览: 35
好的,以下是一个基于图的带权值的邻接矩阵的图的基本操作的演示程序,使用了C语言:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_VERTEX_NUM 100 // 最大顶点数
// 边的定义
typedef struct {
int ivex; // 该边所指向的顶点的位置
int weight; // 权值
} ArcNode;
// 顶点的定义
typedef struct {
char data; // 顶点数据
ArcNode *firstarc; // 指向第一个边节点的指针
} VertexNode;
// 图的定义
typedef struct {
VertexNode vexs[MAX_VERTEX_NUM]; // 顶点数组
int arcnum; // 边数
int vexnum; // 顶点数
} MGraph;
// 创建图
void createGraph(MGraph *G) {
printf("请输入顶点数和边数:\n");
scanf("%d %d", &G->vexnum, &G->arcnum);
// 初始化顶点
for (int i = 0; i < G->vexnum; i++) {
printf("请输入第%d个顶点的数据:\n", i + 1);
scanf(" %c", &G->vexs[i].data);
G->vexs[i].firstarc = NULL;
}
// 初始化边
for (int i = 0; i < G->arcnum; i++) {
int v1, v2, w;
printf("请输入第%d条边所依附的两个顶点及其权值:\n", i + 1);
scanf("%d %d %d", &v1, &v2, &w);
// 创建新的边节点
ArcNode *arcnode = (ArcNode *)malloc(sizeof(ArcNode));
arcnode->ivex = v2 - 1;
arcnode->weight = w;
// 将边节点插入到顶点的边链表中
arcnode->nextarc = G->vexs[v1 - 1].firstarc;
G->vexs[v1 - 1].firstarc = arcnode;
}
}
// 打印图
void printGraph(MGraph G) {
printf("该图的邻接矩阵表示如下:\n");
for (int i = 0; i < G.vexnum; i++) {
for (int j = 0; j < G.vexnum; j++) {
if (G.vexs[i].firstarc == NULL) {
printf("%-6d", 0);
} else {
ArcNode *arcnode = G.vexs[i].firstarc;
bool flag = false; // 判断是否找到该顶点的边
while (arcnode != NULL) {
if (arcnode->ivex == j) {
printf("%-6d", arcnode->weight);
flag = true;
break;
}
arcnode = arcnode->nextarc;
}
if (!flag) {
printf("%-6d", 0);
}
}
}
printf("\n");
}
}
int main() {
MGraph G;
createGraph(&G);
printGraph(G);
return 0;
}
```
该程序实现了基本的图的创建和打印功能,其中使用邻接矩阵来表示图,使用链表来存储每个顶点的边。用户可以输入顶点数和边数,以及每个顶点的数据和每条边的两个顶点及其权值。程序会根据用户输入的数据创建图,并输出邻接矩阵表示的图。
相关推荐
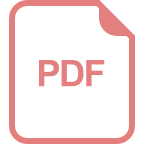
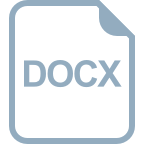
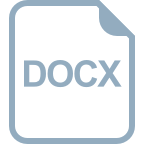














