(1)要求定义一个复数类,利用运算符重载定义该复数类的加法 减法,取反运算。注意双目运算使用友元方式,单目使用成员方式。 另外使用转换函数实现该类对double类型的相互转化。
时间: 2024-03-15 07:44:46 浏览: 76
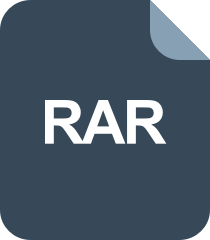
复数运算程序 包括 加法 减法等运算
好的,根据您的要求,我为您提供以下代码实现:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real, imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 运算符重载:加法
friend Complex operator+(const Complex& c1, const Complex& c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
// 运算符重载:减法
friend Complex operator-(const Complex& c1, const Complex& c2) {
return Complex(c1.real - c2.real, c1.imag - c2.imag);
}
// 运算符重载:取反
Complex operator-() const {
return Complex(-real, -imag);
}
// 转换函数:double 转 Complex
operator double() const {
return real;
}
// 转换函数:Complex 转 double
operator Complex() const {
return *this;
}
double getReal() const {
return real;
}
double getImag() const {
return imag;
}
};
int main() {
Complex c1(1, 2), c2(3, 4);
// 加法
Complex c3 = c1 + c2;
cout << c3.getReal() << " + " << c3.getImag() << "i" << endl;
// 减法
Complex c4 = c1 - c2;
cout << c4.getReal() << " + " << c4.getImag() << "i" << endl;
// 取反
Complex c5 = -c1;
cout << c5.getReal() << " + " << c5.getImag() << "i" << endl;
// double 转 Complex
double d = 3.14;
Complex c6 = d;
cout << c6.getReal() << " + " << c6.getImag() << "i" << endl;
// Complex 转 double
Complex c7(1.23);
double d2 = c7;
cout << d2 << endl;
return 0;
}
```
以上代码定义了一个复数类 `Complex`,并实现了加法、减法、取反三个运算符的重载,以及 double 类型和 Complex 类型之间的相互转化。其中,加法和减法使用了友元函数的方式,取反使用了成员函数的方式。同时,转换函数使用了 `operator double() const` 和 `operator Complex() const` 的方式实现。
阅读全文
相关推荐

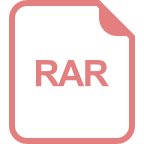
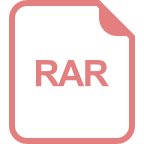









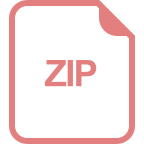