Java用mapreduce 处理
时间: 2023-08-20 14:40:56 浏览: 63
MapReduce是一种分布式计算模型,可以用于处理大规模数据集。在Java中,可以使用Hadoop实现MapReduce计算模型。
MapReduce模型包括两个主要的操作:Map和Reduce。Map操作将输入数据分割成若干个小的数据块,然后对每个数据块进行处理,产生若干个键值对。Reduce操作将相同的键值对进行合并,产生最终的结果。
在Java中,实现MapReduce需要编写Mapper类和Reducer类。Mapper类将输入数据转换成键值对,并输出给Reducer类进行处理。Reducer类将相同的键值对进行合并,产生最终的结果。例如:
```
public class WordCountMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable ONE = new IntWritable(1);
private Text word = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
StringTokenizer tokenizer = new StringTokenizer(line);
while (tokenizer.hasMoreTokens()) {
word.set(tokenizer.nextToken());
context.write(word, ONE);
}
}
}
public class WordCountReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
context.write(key, new IntWritable(sum));
}
}
```
上述代码中,WordCountMapper类将输入数据转换成键值对,其中键为单词,值为1。WordCountReducer类将相同的单词进行合并,并输出最终的结果。
Java使用Hadoop实现MapReduce时,需要将Mapper类和Reducer类打包成jar包,并使用hadoop命令运行。例如:
```
hadoop jar wordcount.jar WordCount input output
```
上述命令将输入文件input进行MapReduce计算,并将结果保存在output文件中。
相关推荐
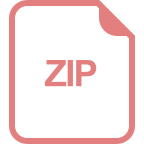
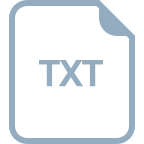
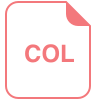
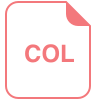
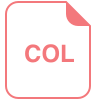
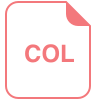
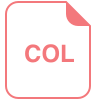









