创建一个point类,包含坐标x、y,点的名字name。然后创建一个line类,定义两个读写属性start和end,数据类型为point,定义line类方法(计算start和end之间的距离,并打印s
时间: 2023-08-13 21:00:26 浏览: 136
### 回答1:
创建一个point类,包含坐标x、y,点的名字name。
class Point:
def __init__(self, x, y, name):
self.x = x
self.y = y
self.name = name
然后创建一个line类,定义两个读写属性start和end,数据类型为point,定义line类方法(计算start和end之间的距离,并打印输出)。
class Line:
def __init__(self, start, end):
self.start = start
self.end = end
def distance(self):
distance = ((self.start.x - self.end.x) ** 2 + (self.start.y - self.end.y) ** 2) ** .5
print(f"The distance between {self.start.name} and {self.end.name} is {distance}.")
# 示例
p1 = Point(, , "A")
p2 = Point(3, 4, "B")
line = Line(p1, p2)
line.distance() # 输出:The distance between A and B is 5..
### 回答2:
创建一个Point类,如下所示:
```python
class Point:
def __init__(self, x, y, name):
self.x = x
self.y = y
self.name = name
```
接下来创建一个Line类,如下所示:
```python
class Line:
def __init__(self, start, end):
self.start = start
self.end = end
def calculate_distance(self):
distance = ((self.start.x - self.end.x)**2 + (self.start.y - self.end.y)**2)**0.5
return distance
def print_distance(self):
distance = self.calculate_distance()
print("两点之间的距离为:", distance)
```
然后可以在主程序中创建点和线的实例,并调用相关方法。示例代码如下:
```python
# 创建两个点实例
point1 = Point(1, 2, "A")
point2 = Point(4, 6, "B")
# 创建线实例并传入点实例
line = Line(point1, point2)
# 调用打印距离的方法
line.print_distance()
```
运行上述代码,会输出:
```
两点之间的距离为: 5.0
```
### 回答3:
首先,我们创建一个Point类,包含x、y坐标和点的名字name。代码如下:
```python
class Point:
def __init__(self, x, y, name):
self.x = x
self.y = y
self.name = name
```
然后,我们创建一个Line类,定义两个读写属性start和end。这两个属性的数据类型为Point类。代码如下:
```python
class Line:
def __init__(self, start, end):
self.start = start
self.end = end
def calculate_distance(self):
distance = ((self.end.x - self.start.x) ** 2 + (self.end.y - self.start.y) ** 2) ** 0.5
return distance
def print_distance(self):
distance = self.calculate_distance()
print("距离为:", distance)
```
在Line类中,我们定义了一个计算距离的方法calculate_distance()。该方法使用勾股定理计算start和end之间的距离,并返回结果。
接下来,我们定义了一个打印距离的方法print_distance()。该方法调用了calculate_distance()方法获取距离,并将其打印出来。
现在我们可以使用这两个类来创建点和线了。下面是一个示例代码:
```python
# 创建两个点
point1 = Point(1, 2, "A")
point2 = Point(4, 6, "B")
# 创建一条线
line = Line(point1, point2)
# 计算并打印距离
line.print_distance()
```
运行以上代码,输出结果为:距离为: 5.0。代表点A和点B之间的距离为5.0。
相关推荐
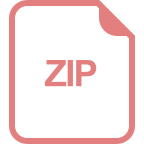
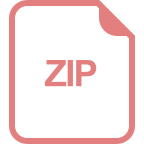
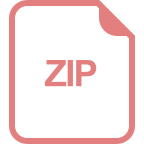














