请提供一个byte[] to hexStr的java方法
时间: 2023-12-07 22:05:40 浏览: 165
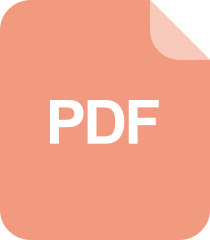
Java中byte[]、String、Hex字符串等转换的方法

可以使用以下的Java方法将byte数组转换为hex字符串:
```java
public static String bytesToHex(byte[] bytes) {
StringBuilder hex = new StringBuilder();
for (int i = 0; i < bytes.length; i++) {
byte b = bytes[i];
boolean negative = false;
if (b < 0) {
negative = true;
b = (byte) (256 + b);
}
int hi = b >> 4;
int lo = b & 0xf;
hex.append((char) (hi < 10 ? '0' + hi : 'a' + hi - 10));
hex.append((char) (lo < 10 ? '0' + lo : 'a' + lo - 10));
}
return hex.toString();
}
```
这个方法会将byte数组中的每个元素转换为16进制表示的字符串,并将它们拼接在一起返回一个完整的hex字符串。
阅读全文
相关推荐
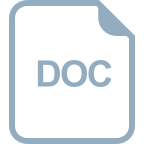
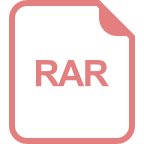
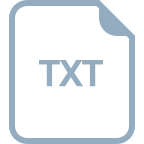
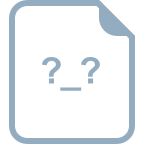
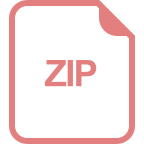
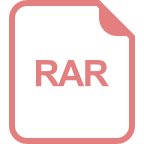
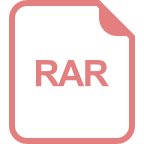
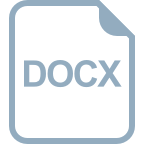
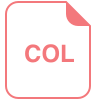
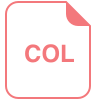






