手写数字识别的手写板界面设计,通过手写板进行识别并将效果显示在界面上,同时手写板输入的张量要满足形状为 (None, 10) 的占位符的模型,调用pb格式的模型
时间: 2024-05-03 20:18:39 浏览: 13
手写数字识别的手写板界面设计可以使用 Python 的 Tkinter 模块来实现。以下是一个简单的示例代码:
```python
import tkinter as tk
import tensorflow as tf
import numpy as np
# 加载模型
model_path = 'path/to/model.pb'
model = tf.compat.v1.keras.models.load_model(model_path)
# 创建手写板界面
class HandwritingRecognition(tk.Canvas):
def __init__(self, master=None, width=280, height=280):
super().__init__(master, width=width, height=height, bg='white')
self.master = master
self.width = width
self.height = height
self.bind('<B1-Motion>', self.draw)
def draw(self, event):
x, y = event.x, event.y
r = 8
self.create_oval(x-r, y-r, x+r, y+r, fill='black')
def recognize(self):
# 将手写板图片转换成模型需要的张量
img = self.postscript(colormode='gray')
img = np.fromstring(img.encode('utf-8'), dtype=np.uint8, sep=' ')
img = img.reshape(self.height, self.width)
img = img / 255.0
img = np.expand_dims(img, axis=0)
img = np.expand_dims(img, axis=-1)
# 使用模型进行预测
pred = model.predict(img)
pred = np.argmax(pred, axis=1)
# 在界面上显示预测结果
self.delete('all')
self.create_text(self.width/2, self.height/2, text=str(pred[0]), font=('Arial', 120))
# 创建主界面
root = tk.Tk()
root.title('Handwriting Recognition')
root.geometry('300x370')
# 创建手写板和按钮
canvas = HandwritingRecognition(root)
canvas.pack(fill=tk.BOTH, expand=True)
button = tk.Button(root, text='Recognize', command=canvas.recognize)
button.pack(side=tk.BOTTOM, pady=5)
# 运行主程序
root.mainloop()
```
在这个示例代码中,我们首先加载了一个 pb 格式的模型,并创建了一个 HandwritingRecognition 类来实现手写板界面。在界面上,用户可以使用鼠标在手写板上绘制数字,然后点击“Recognize”按钮来识别绘制的数字。在 HandwritingRecognition 类的 recognize 方法中,我们首先将手写板图片转换成模型需要的张量,然后使用模型进行预测,并在界面上显示预测结果。需要注意的是,我们在模型中使用了一个形状为 (None, 10) 的占位符,其中 None 表示可变长度,因此我们在代码中使用了 np.expand_dims 将输入张量的形状转换成 (1, 280, 280, 1)。
相关推荐
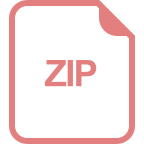
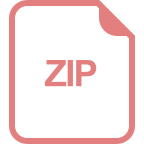
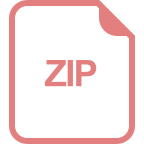














