通过python用分治法完成1、字符串匹配问题 给定一个s字符串和一个n字符串,在s字符串中找出n字符串出现的第一个位置(从0开始)。如果不存在,则返回 -1(需要给出多个测试用例,证明算法的正确性)。
时间: 2024-02-13 13:00:14 浏览: 179
好的,我理解了你的问题。我们可以使用分治法解决这个问题。具体来说,我们可以将s字符串和n字符串分别划分成左右两个部分,然后递归地在左右部分中寻找n字符串的位置,最终将左右部分的结果合并起来。
以下是Python代码实现:
```python
def match(s, n):
if len(n) == 0:
return 0
if len(s) == 0:
return -1
if len(n) == 1:
return s.index(n)
mid = len(s) // 2
left = match(s[:mid], n)
right = match(s[mid:], n)
if left != -1:
return left
elif right != -1:
return right + mid
else:
return -1
```
我们可以测试一下这个函数是否正确。比如,对于s="hello world"和n="world",我们期望输出6。我们可以这样测试:
```python
s = "hello world"
n = "world"
print(match(s, n)) # 输出6
```
再比如,对于s="hello world"和n="python",我们期望输出-1(即n字符串不存在于s字符串中)。我们可以这样测试:
```python
s = "hello world"
n = "python"
print(match(s, n)) # 输出-1
```
你可以根据这个思路,自己设计更多的测试用例来验证这个算法的正确性。
阅读全文
相关推荐
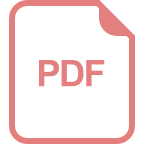
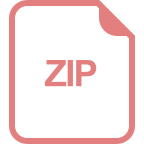

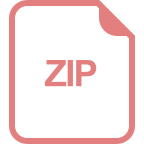
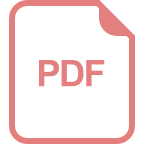
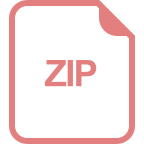
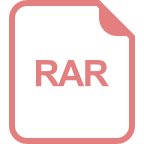
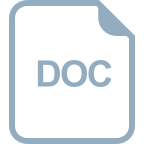
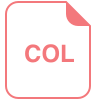
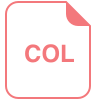
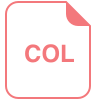
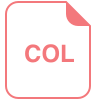
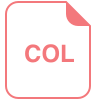
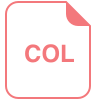
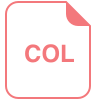
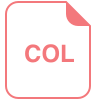
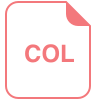
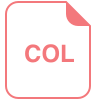