java读取指定sheet页数据
时间: 2023-08-07 11:08:35 浏览: 172
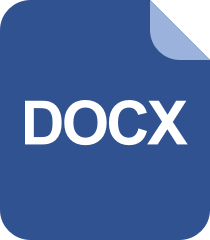
java如何读取Excel中的数据

要在 Java 中读取指定的 Excel 工作表(sheet)的数据,您可以使用 Apache POI 库。以下是一个示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
public class ExcelReader {
public static void main(String[] args) {
String filePath = "path/to/your/excel/file.xlsx";
String sheetName = "Sheet1";
try (FileInputStream fis = new FileInputStream(filePath);
Workbook workbook = new XSSFWorkbook(fis)) {
Sheet sheet = workbook.getSheet(sheetName);
if (sheet == null) {
System.out.println("Sheet not found: " + sheetName);
return;
}
for (Row row : sheet) {
for (Cell cell : row) {
CellType cellType = cell.getCellType();
if (cellType == CellType.STRING) {
System.out.print(cell.getStringCellValue() + "\t");
} else if (cellType == CellType.NUMERIC) {
System.out.print(cell.getNumericCellValue() + "\t");
} else if (cellType == CellType.BOOLEAN) {
System.out.print(cell.getBooleanCellValue() + "\t");
} else if (cellType == CellType.BLANK) {
System.out.print("\t");
}
}
System.out.println();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
您需要将 `filePath` 替换为您的 Excel 文件路径,`sheetName` 替换为您要读取的工作表名称。上述代码使用 `XSSFWorkbook` 类来读取 `.xlsx` 格式的文件,如果您要读取 `.xls` 格式的文件,可以使用 `HSSFWorkbook` 类。
代码将逐行打印工作表中的数据,每个单元格之间用制表符分隔。您可以根据需要对代码进行修改,以满足您的具体要求。
希望这个示例代码能帮助到您。如果您有其他问题,请随时提问。
阅读全文
相关推荐
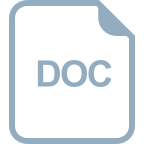
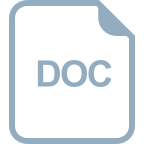


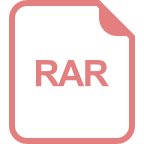
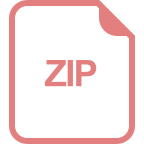
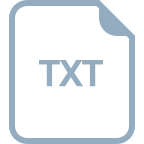
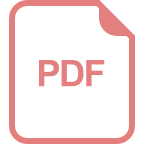
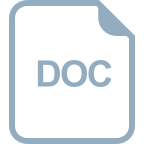
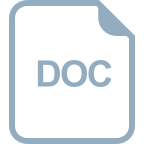







