编写创建进程队列的程序段和进程调度函数,用C编译
时间: 2024-02-13 20:02:51 浏览: 23
以下是一个简单的创建进程队列的程序段和进程调度函数的示例,使用C语言编写:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义进程控制块结构体
typedef struct pcb {
int pid; // 进程ID
int priority; // 进程优先级
struct pcb *next; // 指向下一个进程控制块的指针
} PCB;
// 定义进程队列结构体
typedef struct process_queue {
PCB *head; // 队列头指针
PCB *tail; // 队列尾指针
} ProcessQueue;
// 创建一个空的进程队列
ProcessQueue *create_process_queue() {
ProcessQueue *queue = (ProcessQueue*) malloc(sizeof(ProcessQueue));
queue->head = NULL;
queue->tail = NULL;
return queue;
}
// 添加一个进程控制块到进程队列中
void enqueue_process(ProcessQueue *queue, int pid, int priority) {
PCB *new_pcb = (PCB*) malloc(sizeof(PCB));
new_pcb->pid = pid;
new_pcb->priority = priority;
new_pcb->next = NULL;
if (queue->head == NULL) {
queue->head = new_pcb;
queue->tail = new_pcb;
} else {
queue->tail->next = new_pcb;
queue->tail = new_pcb;
}
}
// 从进程队列中移除一个进程控制块
void dequeue_process(ProcessQueue *queue) {
if (queue->head == NULL) {
printf("队列已空,无法出队!\n");
return;
}
PCB *temp = queue->head;
queue->head = temp->next;
if (queue->head == NULL) {
queue->tail = NULL;
}
free(temp);
}
// 进程调度函数,返回队列中优先级最高的进程ID
int schedule_process(ProcessQueue *queue) {
if (queue->head == NULL) {
printf("队列为空,无法进行调度!\n");
return -1;
}
PCB *temp = queue->head;
int max_priority = temp->priority;
int max_pid = temp->pid;
while (temp != NULL) {
if (temp->priority > max_priority) {
max_priority = temp->priority;
max_pid = temp->pid;
}
temp = temp->next;
}
return max_pid;
}
int main() {
ProcessQueue *queue = create_process_queue();
enqueue_process(queue, 1, 3);
enqueue_process(queue, 2, 5);
enqueue_process(queue, 3, 1);
printf("队列中优先级最高的进程ID为:%d\n", schedule_process(queue));
dequeue_process(queue);
printf("队列中优先级最高的进程ID为:%d\n", schedule_process(queue));
return 0;
}
```
注意,在这个示例中,我们使用了链表来实现进程队列。进程控制块(PCB)结构体中包含了进程ID、进程优先级和指向下一个进程控制块的指针。进程队列结构体中包含了队列头指针和队列尾指针。
我们创建了三个进程控制块,并将它们添加到队列中。然后,我们使用进程调度函数来获取队列中优先级最高的进程ID。最后,我们将队列中的第一个进程控制块(即优先级最高的进程控制块)移除队列。再次调用进程调度函数,我们可以看到队列中新的优先级最高的进程ID。
相关推荐
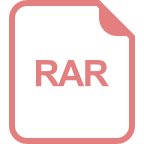
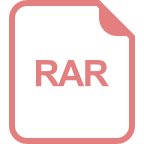
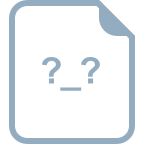














