使用c语言编写实现两个进程实现消息队列通信的程序
时间: 2023-06-18 08:04:33 浏览: 79
以下是使用C语言编写的两个进程实现消息队列通信的示例程序:
进程1:发送消息
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSGSZ 128
typedef struct msgbuf {
long mtype;
char mtext[MSGSZ];
} message_buf;
int main()
{
int msqid;
key_t key;
message_buf sbuf;
size_t buflen;
// 创建消息队列
key = ftok(".", 'a');
msqid = msgget(key, 0666 | IPC_CREAT);
if (msqid == -1) {
perror("msgget");
exit(1);
}
// 准备发送的消息
sbuf.mtype = 1;
strcpy(sbuf.mtext, "Hello world!");
buflen = strlen(sbuf.mtext) + 1;
// 发送消息
if (msgsnd(msqid, &sbuf, buflen, IPC_NOWAIT) == -1) {
perror("msgsnd");
exit(1);
}
printf("Sent message: %s\n", sbuf.mtext);
return 0;
}
```
进程2:接收消息
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSGSZ 128
typedef struct msgbuf {
long mtype;
char mtext[MSGSZ];
} message_buf;
int main()
{
int msqid;
key_t key;
message_buf rbuf;
size_t buflen;
// 获取消息队列
key = ftok(".", 'a');
msqid = msgget(key, 0666 | IPC_CREAT);
if (msqid == -1) {
perror("msgget");
exit(1);
}
// 接收消息
if (msgrcv(msqid, &rbuf, MSGSZ, 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
buflen = strlen(rbuf.mtext) + 1;
printf("Received message: %s\n", rbuf.mtext);
// 删除消息队列
if (msgctl(msqid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
以上程序中,进程1发送了一条消息到消息队列中,进程2接收了这条消息并打印出来。在实际使用中,可以根据需要修改消息类型、消息内容和消息长度等参数。
相关推荐
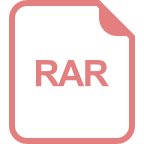
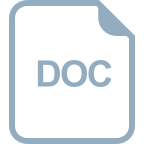
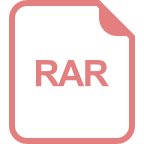














