C语言实现消息队列的收发
时间: 2024-02-05 09:54:51 浏览: 102
C语言中可以使用System V IPC或POSIX IPC实现消息队列的收发。
使用System V IPC,可以按照以下步骤进行:
1. 创建消息队列:使用msgget函数创建一个消息队列,指定队列的键值和一些选项。
2. 发送消息:使用msgsnd函数向消息队列中发送消息,指定队列的标识符、消息类型和消息数据。
3. 接收消息:使用msgrcv函数从消息队列中接收消息,指定队列的标识符、期望接收的消息类型和接收缓冲区的大小。
4. 删除消息队列:使用msgctl函数删除消息队列,指定队列的标识符和一些选项。
使用POSIX IPC,可以按照以下步骤进行:
1. 创建消息队列:使用mq_open函数创建一个消息队列,指定队列的名称和一些选项。
2. 发送消息:使用mq_send函数向消息队列中发送消息,指定队列的描述符、消息数据和消息大小。
3. 接收消息:使用mq_receive函数从消息队列中接收消息,指定队列的描述符、接收缓冲区的大小和用于存储消息类型的指针。
4. 删除消息队列:使用mq_unlink函数删除消息队列,指定队列的名称。
相关问题
C语言实现消息队列的收发的例子
以下是一个使用System V IPC实现消息队列收发的示例程序:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_SIZE 1024
// 定义消息结构体
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
key_t key;
int msgid;
struct msgbuf msg;
// 生成一个唯一的键值
key = ftok(".", 'q');
if (key == -1) {
perror("ftok");
exit(EXIT_FAILURE);
}
// 创建消息队列
msgid = msgget(key, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 发送消息
msg.mtype = 1;
strcpy(msg.mtext, "Hello, world!");
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
// 接收消息
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 0, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
printf("Received message: %s\n", msg.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
return 0;
}
```
以下是一个使用POSIX IPC实现消息队列收发的示例程序:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <mqueue.h>
#define MSG_SIZE 1024
#define QUEUE_NAME "/my_queue"
int main() {
mqd_t mq;
struct mq_attr attr;
char buffer[MSG_SIZE + 1];
int msg_len;
// 设置消息队列的属性
attr.mq_flags = 0;
attr.mq_maxmsg = 10;
attr.mq_msgsize = MSG_SIZE;
attr.mq_curmsgs = 0;
// 创建消息队列
mq = mq_open(QUEUE_NAME, O_CREAT | O_RDWR, 0666, &attr);
if (mq == (mqd_t) -1) {
perror("mq_open");
exit(EXIT_FAILURE);
}
// 发送消息
if (mq_send(mq, "Hello, world!", MSG_SIZE, 0) == -1) {
perror("mq_send");
exit(EXIT_FAILURE);
}
// 接收消息
msg_len = mq_receive(mq, buffer, MSG_SIZE, NULL);
if (msg_len == -1) {
perror("mq_receive");
exit(EXIT_FAILURE);
}
buffer[msg_len] = '\0';
printf("Received message: %s\n", buffer);
// 删除消息队列
if (mq_close(mq) == -1) {
perror("mq_close");
exit(EXIT_FAILURE);
}
if (mq_unlink(QUEUE_NAME) == -1) {
perror("mq_unlink");
exit(EXIT_FAILURE);
}
return 0;
}
```
请给出C语言 IPC消息队列 收发例子,并给出主要函数原型和参数说明
下面是一个简单的C语言IPC消息队列收发例子:
发送消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAXSIZE 128
struct msgbuf
{
long mtype;
char mtext[MAXSIZE];
};
int main()
{
key_t key;
int msgid;
struct msgbuf msg;
key = ftok(".", 'a'); // 创建key值
msgid = msgget(key, IPC_CREAT|0666); // 创建消息队列
msg.mtype = 1; // 设置消息类型
strcpy(msg.mtext, "Hello, world!"); // 设置消息内容
msgsnd(msgid, &msg, strlen(msg.mtext)+1, 0); // 发送消息
printf("Send message: %s\n", msg.mtext);
exit(0);
}
```
主要函数原型和参数说明:
```c
key_t ftok(const char *pathname, int proj_id); // 创建key值
int msgget(key_t key, int msgflg); // 创建或打开消息队列
// key:消息队列的key值,msgflg:权限标志和标记
ssize_t msgrcv(int msgid, void *msgp, size_t msgsz, long msgtyp, int msgflg); // 接收消息
// msgid:消息队列的ID,msgp:存储消息的缓冲区,msgsz:消息缓冲区的大小,msgtyp:消息类型,msgflg:权限标志和标记
int msgsnd(int msgid, const void *msgp, size_t msgsz, int msgflg); // 发送消息
// msgid:消息队列的ID,msgp:存储消息的缓冲区,msgsz:消息缓冲区的大小,msgflg:权限标志和标记
```
接收消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAXSIZE 128
struct msgbuf
{
long mtype;
char mtext[MAXSIZE];
};
int main()
{
key_t key;
int msgid;
struct msgbuf msg;
key = ftok(".", 'a'); // 创建key值
msgid = msgget(key, IPC_CREAT|0666); // 创建消息队列
msgrcv(msgid, &msg, MAXSIZE, 1, 0); // 接收消息
printf("Receive message: %s\n", msg.mtext);
exit(0);
}
```
主要函数原型和参数说明与发送消息相同。
阅读全文
相关推荐
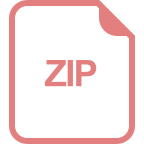
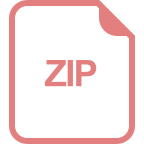
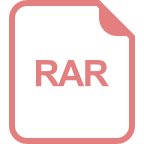









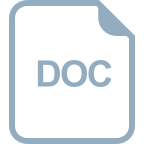
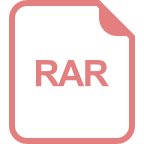
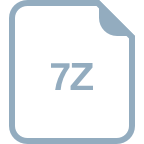
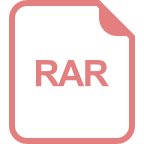
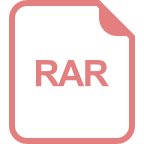